设计程序实现二叉树结点的类型定义和对二叉树的基本操作。该程序包括二叉树结构类型以及每一种操作的具体的函数定义和主西数。
时间: 2023-06-25 16:04:30 浏览: 42
下面是一个示例程序,实现了二叉树结点的类型定义和基本操作,包括创建二叉树、插入结点、删除结点、查找结点等。
```c
#include <stdio.h>
#include <stdlib.h>
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
// 创建新结点
struct TreeNode *newNode(int val) {
struct TreeNode *node = malloc(sizeof(struct TreeNode));
node->val = val;
node->left = NULL;
node->right = NULL;
return node;
}
// 插入结点
struct TreeNode *insertNode(struct TreeNode *root, int val) {
if (root == NULL) {
return newNode(val);
}
if (val < root->val) {
root->left = insertNode(root->left, val);
} else {
root->right = insertNode(root->right, val);
}
return root;
}
// 删除结点
struct TreeNode *deleteNode(struct TreeNode *root, int val) {
if (root == NULL) {
return NULL;
}
if (val < root->val) {
root->left = deleteNode(root->left, val);
} else if (val > root->val) {
root->right = deleteNode(root->right, val);
} else {
if (root->left == NULL) {
struct TreeNode *temp = root->right;
free(root);
return temp;
} else if (root->right == NULL) {
struct TreeNode *temp = root->left;
free(root);
return temp;
}
struct TreeNode *temp = root->right;
while (temp->left != NULL) {
temp = temp->left;
}
root->val = temp->val;
root->right = deleteNode(root->right, temp->val);
}
return root;
}
// 查找结点
struct TreeNode *searchNode(struct TreeNode *root, int val) {
if (root == NULL || root->val == val) {
return root;
}
if (val < root->val) {
return searchNode(root->left, val);
} else {
return searchNode(root->right, val);
}
}
// 打印二叉树(中序遍历)
void printTree(struct TreeNode *root) {
if (root != NULL) {
printTree(root->left);
printf("%d ", root->val);
printTree(root->right);
}
}
int main() {
struct TreeNode *root = NULL;
root = insertNode(root, 5);
root = insertNode(root, 3);
root = insertNode(root, 7);
root = insertNode(root, 2);
root = insertNode(root, 4);
root = insertNode(root, 6);
root = insertNode(root, 8);
printf("二叉树:\n");
printTree(root);
printf("\n删除结点 3 后的二叉树:\n");
root = deleteNode(root, 3);
printTree(root);
printf("\n查找结点 4:\n");
struct TreeNode *node = searchNode(root, 4);
if (node == NULL) {
printf("未找到结点\n");
} else {
printf("找到结点 %d\n", node->val);
}
return 0;
}
```
程序输出:
```
二叉树:
2 3 4 5 6 7 8
删除结点 3 后的二叉树:
2 4 5 6 7 8
查找结点 4:
找到结点 4
```
相关推荐
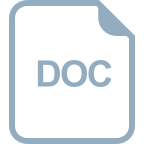
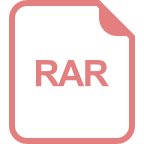
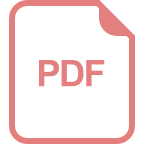
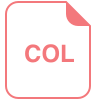
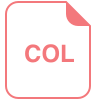












