遍历dataframe的每一行元素
时间: 2023-08-04 11:17:50 浏览: 142
可以`iterrows()`方法来遍历DataFrame的每一行元素,示例代码如下:
```python
import pandas as pd
# 创建一个DataFrame
df = pd.DataFrame({'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 35]})
# 使用iterrows()方法遍历每一行元素
for index, row in df.iterrows():
print(index, row['name'], row['age'])
```
输出结果为:
```
0 Alice 25
1 Bob 30
2 Charlie 35
```
其中`index`表示行索引,`row['name']`和`row['age']`分别表示该行`name`和`age`列的值。
相关问题
python for循环遍历dataframe每行
在Python中,使用for循环遍历DataFrame中的每一行可以使用iterrows()方法。iterrows()方法将DataFrame中的每一行表示为一个元组(index, Series),其中index是行索引,Series是该行数据。
首先,导入pandas库并创建一个DataFrame:
```python
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]})
```
然后,可以使用iterrows()方法迭代每一行并处理数据:
```python
for index, row in df.iterrows():
print(row['A'], row['B'], row['C'])
```
在上面的代码中,每次迭代都会处理一行数据。因为row是一个Series对象,可以通过列名索引直接访问每个元素的值。
另外,需要注意的是,由于iterrows()方法需要逐行迭代DataFrame,所以在处理大型数据集时可能会比较慢。如果需要更高效的方法,可以使用其他一些方法,例如iteritems()或itertuples()。
如何遍历dataframe的行
可以使用`iterrows()`函数来遍历DataFrame的每一行。这个函数返回一个迭代器,每个元素都是一个元组,包含行的索引和行的数据。
下面是一个简单的示例代码:
```python
import pandas as pd
# 创建一个DataFrame
df = pd.DataFrame({'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 35]})
# 遍历每一行
for index, row in df.iterrows():
print(f"Name: {row['name']}, Age: {row['age']}")
```
输出:
```
Name: Alice, Age: 25
Name: Bob, Age: 30
Name: Charlie, Age: 35
```
在每次循环中,`iterrows()`返回一个元组,元组的第一个元素是行的索引,第二个元素是包含该行数据的Series对象。你可以使用`row['column_name']`来访问每个列的值。
阅读全文
相关推荐
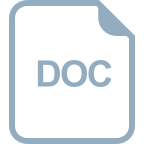
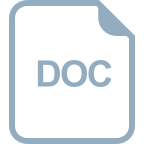
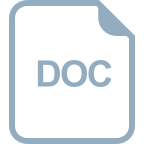
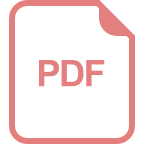
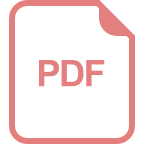
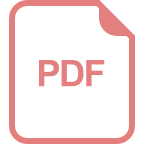










