pytorch实现CutMix数据增强和正则交叉熵损失函数调用
时间: 2023-11-02 12:11:50 浏览: 135
CutMix是一种数据增强技术,可以在训练神经网络时,将两个不同的图像混合在一起,生成一个新的图像。这种技术可以增加模型的鲁棒性和泛化能力。
以下是使用PyTorch实现CutMix数据增强的代码:
```python
import torch
import numpy as np
import random
def cutmix_data(x, y, alpha=1.0):
lam = np.random.beta(alpha, alpha)
batch_size = x.size()[0]
index = torch.randperm(batch_size)
y_a, y_b = y, y[index]
bbx1, bby1, bbx2, bby2 = rand_bbox(x.size(), lam)
x[:, :, bbx1:bbx2, bby1:bby2] = x[index, :, bbx1:bbx2, bby1:bby2]
lam = 1 - ((bbx2 - bbx1) * (bby2 - bby1) / (x.size()[-1] * x.size()[-2]))
return x, y_a, y_b, lam
def rand_bbox(size, lam):
W = size[2]
H = size[3]
cut_rat = np.sqrt(1. - lam)
cut_w = np.int(W * cut_rat)
cut_h = np.int(H * cut_rat)
# uniform
cx = np.random.randint(W)
cy = np.random.randint(H)
bbx1 = np.clip(cx - cut_w // 2, 0, W)
bby1 = np.clip(cy - cut_h // 2, 0, H)
bbx2 = np.clip(cx + cut_w // 2, 0, W)
bby2 = np.clip(cy + cut_h // 2, 0, H)
return bbx1, bby1, bbx2, bby2
```
正则交叉熵损失函数是一种可以减少标签噪声对模型训练的影响的损失函数。以下是使用PyTorch实现正则交叉熵损失函数的代码:
```python
import torch.nn.functional as F
def reg_cross_entropy_loss(input, target, weight=None, size_average=None,
ignore_index=-100, reduce=None, reduction='mean',
reg_lambda=0.1):
logp = F.log_softmax(input, dim=1)
loss = F.nll_loss(logp, target, weight, size_average, ignore_index, reduce, reduction)
reg_loss = torch.mean(torch.sum(torch.square(torch.exp(logp)), dim=1))
return loss + reg_lambda * reg_loss
```
在调用此函数时,您可以指定reg_lambda参数来控制正则化的程度。
阅读全文
相关推荐
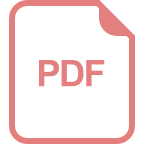
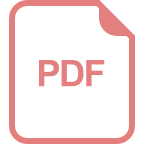
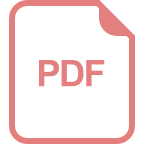
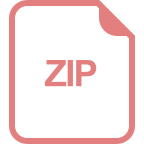

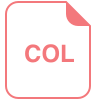

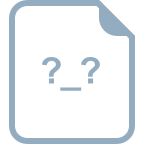
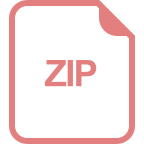
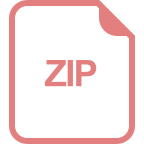
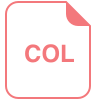
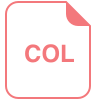
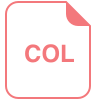
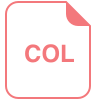




