使用【python】写实验四 1、url='https://www.weather.com.cn/weather/101040100.shtml',从重庆天气网站上爬取最近7天的最高温和最低温,绘制出如下的曲线图
时间: 2024-12-23 07:23:00 浏览: 6
在Python中进行这个任务,你需要使用一些库,比如requests获取网页内容,BeautifulSoup解析HTML,pandas处理数据,matplotlib绘制图表。以下是简单的步骤:
首先,安装必要的库(如果你还没有安装的话):
```bash
pip install requests beautifulsoup4 pandas matplotlib
```
然后,你可以编写如下代码:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
import matplotlib.pyplot as plt
# Step 1: 获取网页内容
url = 'https://www.weather.com.cn/weather/101040100.shtml'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Step 2: 解析数据
data = []
table = soup.find('div', {'class': 'list fl mt5'})
rows = table.find_all('tr')
for row in rows[1:]: # 跳过表头
cols = row.find_all('td')
temps = [col.text.strip() for col in cols[-2:]] # 最高温和最低温通常在最后一列
data.append([cols[0].text.strip(), temps[0], temps[1]])
# Step 3: 转换为DataFrame并处理数据
df = pd.DataFrame(data, columns=['日期', '最高温度', '最低温度'])
df['日期'] = pd.to_datetime(df['日期']) # 将日期转换为datetime格式
# Step 4: 绘制曲线图
df.set_index('日期', inplace=True) # 设置日期为索引
plt.figure(figsize=(10,6))
plt.plot(df['最高温度'], label='最高温度')
plt.plot(df['最低温度'], label='最低温度')
plt.title('重庆最近7天最高温和最低气温变化')
plt.xlabel('日期')
plt.ylabel('温度(℃)')
plt.legend()
plt.show()
```
注意:实际操作中可能会遇到网页结构的变化导致解析困难,或者需要处理异常情况。此外,有些网站可能有反爬虫策略,如需要登录或者限制请求频率,这在上述代码中未做处理。
阅读全文
相关推荐
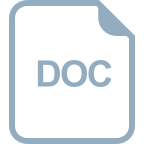
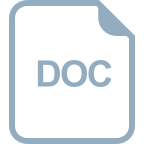
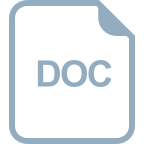
















