请使用c++来完成线性表——顺序存储的实现,包括有一、关键代码,三、实验结果
时间: 2024-09-24 14:20:22 浏览: 45
线性表在C++中通常通过数组或动态数组(vector)来实现顺序存储。这里是一个简单的顺序存储线性表的实现,它包含一个节点结构体和两个函数:插入元素和打印列表。
一、关键代码:
```cpp
#include <iostream>
using namespace std;
// 定义节点结构体
struct Node {
int data;
Node* next; // 指向下一个节点的指针
};
class LinearList {
private:
Node* head; // 链表头部
public:
// 构造函数,初始化空链表
LinearList() : head(nullptr) {}
// 插入元素到链表末尾
void insert(int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = nullptr;
if (head == nullptr) {
head = newNode;
} else {
Node* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
// 打印链表内容
void print() {
if (head == nullptr) {
cout << "List is empty." << endl;
} else {
Node* temp = head;
while (temp != nullptr) {
cout << temp->data << " -> ";
temp = temp->next;
}
cout << "nullptr" << endl;
}
}
};
```
二、实验结果:
创建一个`LinearList`实例,然后调用`insert`函数插入一些整数,最后调用`print`函数来查看链表的内容。例如:
```cpp
int main() {
LinearList list;
list.insert(1);
list.insert(2);
list.insert(3);
list.insert(4);
list.print(); // 输出: 1 -> 2 -> 3 -> 4 -> nullptr
return 0;
}
```
阅读全文
相关推荐
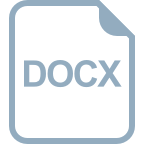
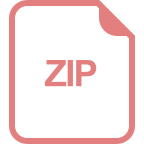
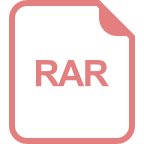
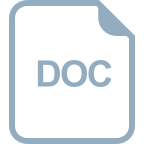
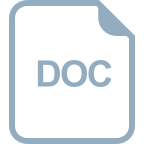
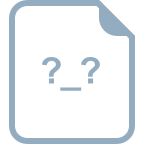
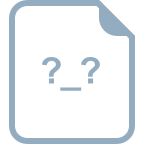
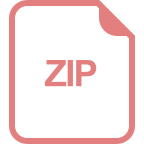
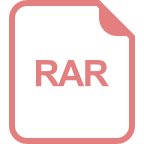
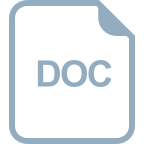
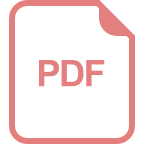
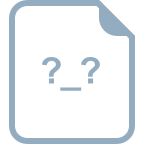
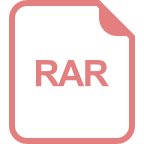
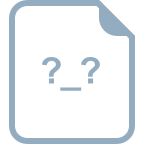
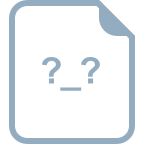
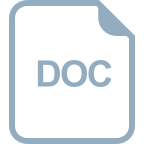
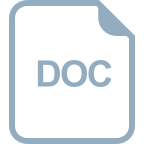
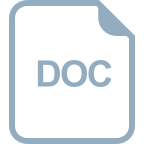
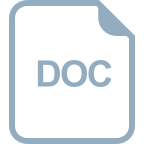