1.设二叉树采用二叉链表存储,编写函数,对二叉树中每个元素值为X的结点,删除以它为根的子树,并释放相应空间。 2.假设二叉树采用二叉链表存储结构,求二叉树b中结点值为x的结点的层次(或深度)
时间: 2024-12-01 17:05:51 浏览: 9
1. 要编写一个删除二叉树中所有值为 X 的节点的函数,首先需要遍历整个二叉链表树结构。这个过程可以递归地完成,从根节点开始搜索:
```python
def delete_nodes(root, value):
if not root:
return None
# 如果当前节点就是要删除的节点,替换为NULL
if root.value == value:
root = None
else:
# 递归处理左子树和右子树
root.left = delete_nodes(root.left, value)
root.right = delete_nodes(root.right, value)
return root
```
然后,对于释放空间的问题,由于二叉链表的节点通常包含指向前驱和后继节点的引用,所以在删除节点后,记得将它们的前驱或后继指向NULL,避免形成悬挂指针。
2. 求解二叉树中结点值为 x 的节点的层次,我们可以使用队列辅助的广度优先搜索(BFS)算法:
```python
def get_depth(node, target_value, current_level=0):
if not node:
return -1
if node.value == target_value:
return current_level
left_depth = get_depth(node.left, target_value, current_level + 1)
right_depth = get_depth(node.right, target_value, current_level + 1)
return max(left_depth, right_depth) if left_depth != -1 or right_depth != -1 else -1
# 使用时,传入二叉树的根节点和目标值即可
depth = get_depth(binary_tree_root, x)
```
阅读全文
相关推荐
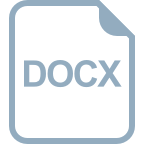
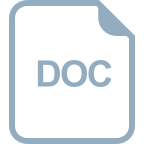
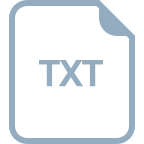















