编写Java程序,定义节点类,实现单向链表的输出、增加、删除和查找等功能。
时间: 2024-02-20 18:01:54 浏览: 18
以下一个示例程序,实现了节点类的定义,以及单向链表的增加、删除、查找和输出等功能:
```java
public class Node {
private int data; // 节点存储的数据
private Node next; // 下一个节点
// 构造函数,初始化节点
public Node(int data) {
this.data = data;
this.next = null;
}
// 获取节点存储的数据
public int getData() {
return data;
}
// 获取下一个节点
public Node getNext() {
return next;
}
// 设置下一个节点
public void setNext(Node next) {
this.next = next;
}
}
public class LinkedList {
private Node head; // 链表头节点
// 构造函数,初始化链表
public LinkedList() {
head = null;
}
// 在链表尾部添加节点
public void add(int data) {
Node newNode = new Node(data);
// 如果链表为空,直接将新节点设置为头节点
if (head == null) {
head = newNode;
return;
}
// 遍历链表,找到尾节点,并将新节点添加到其后面
Node curr = head;
while (curr.getNext() != null) {
curr = curr.getNext();
}
curr.setNext(newNode);
}
// 在链表中删除指定数据的节点
public void remove(int data) {
// 如果链表为空,直接返回
if (head == null) {
return;
}
// 如果头节点就是要删除的节点,直接将头节点指向下一个节点
if (head.getData() == data) {
head = head.getNext();
return;
}
// 遍历链表,查找要删除的节点,并将其从链表中删除
Node prev = head;
Node curr = head.getNext();
while (curr != null) {
if (curr.getData() == data) {
prev.setNext(curr.getNext());
return;
}
prev = curr;
curr = curr.getNext();
}
}
// 在链表中查找指定数据的节点,并返回其在链表中的位置(从1开始)
public int find(int data) {
// 如果链表为空,直接返回-1
if (head == null) {
return -1;
}
// 遍历链表,查找指定数据的节点,并返回其位置
int pos = 1;
Node curr = head;
while (curr != null) {
if (curr.getData() == data) {
return pos;
}
pos++;
curr = curr.getNext();
}
// 如果未找到指定数据的节点,返回-1
return -1;
}
// 输出链表中的所有节点数据
public void printList() {
Node curr = head;
while (curr != null) {
System.out.print(curr.getData() + " ");
curr = curr.getNext();
}
System.out.println();
}
}
```
你可以在 main 函数中创建一个 LinkedList 对象,并调用其方法来测试上述实现。例如:
```java
public static void main(String[] args) {
LinkedList list = new LinkedList();
list.add(1);
list.add(2);
list.add(3);
list.add(4);
list.add(5);
list.printList(); // 输出:1 2 3 4 5
list.remove(3);
list.printList(); // 输出:1 2 4 5
int pos = list.find(4);
System.out.println(pos); // 输出:3
}
```
相关推荐
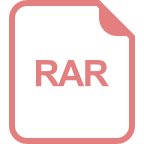
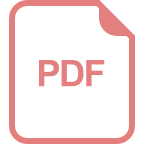
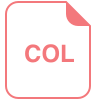













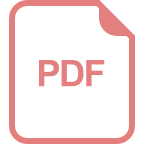