library IEEE; use IEEE.STD_LOGIC_1164.ALL; USE IEEE.STD_LOGIC_UNSIGNED.ALL; entity counter is port( clk : in std_logic; rst ,en: in std_logic; dcnt1: out std_logic_vector(3 downto 0); dcnt2: out std_logic_vector(3 downto 0); add:out std_logic ); end counter; architecture Behavioral of counter is signal cnt10 : std_logic_vector(3 downto 0):="0000"; signal cnt6 : std_logic_vector(3 downto 0):="0000"; begin process(clk,en,rst) begin if (clk'event and clk = '1') then if (rst = '0') then cnt10 <= "0000"; cnt6 <= "0000"; add<='0'; elsif (en='1') then if (cnt10 = "1001") then cnt10<="0000"; cnt6<=cnt6+'1'; else cnt10<=cnt10+'1'; if cnt10="1001"and cnt6="0101" then cnt6<="0000"; cnt10<="0000"; end if; end if; end if; end if; end process ; process(cnt6,cnt10,clk) begin IF clk'EVENT AND clk='1' then if cnt10="1001" and cnt6="0101" then add<='1'; else add<='0'; end if; end if; end process; dcnt1<=cnt6; dcnt2<=cnt10; end Behavioral;这段代码怎么改正
时间: 2024-01-07 17:05:46 浏览: 32
这段代码存在几个问题,需要进行改正:
1. 输出 add 的逻辑不正确,应该在第一个进程中进行计算,而不是在第二个进程中。
2. 在第二个进程中,没有加上 `rst` 的判断,会导致计数器在复位后仍然保留上一次计数的值。
3. 在第二个进程中,使用了 `+` 运算符,但是 `std_logic_vector` 类型不能直接进行加法运算,需要使用 `unsigned` 类型进行转换。
下面是改正后的代码:
```
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
USE IEEE.STD_LOGIC_UNSIGNED.ALL;
entity counter is
port(
clk : in std_logic;
rst, en : in std_logic;
dcnt1 : out std_logic_vector(3 downto 0);
dcnt2 : out std_logic_vector(3 downto 0);
add : out std_logic
);
end counter;
architecture Behavioral of counter is
signal cnt10 : std_logic_vector(3 downto 0) := "0000";
signal cnt6 : std_logic_vector(3 downto 0) := "0000";
begin
process(clk, rst)
begin
if (rst = '0') then
cnt10 <= "0000";
cnt6 <= "0000";
add <= '0';
elsif (clk'event and clk = '1') then
if (en = '1') then
if (cnt10 = "1001") then
cnt10 <= "0000";
cnt6 <= cnt6 + 1;
else
cnt10 <= cnt10 + 1;
if (cnt10 = "1001" and cnt6 = "0101") then
cnt6 <= "0000";
cnt10 <= "0000";
end if;
end if;
if (cnt10 = "1001" and cnt6 = "0101") then
add <= '1';
else
add <= '0';
end if;
end if;
end if;
end process;
dcnt1 <= cnt6;
dcnt2 <= cnt10;
end Behavioral;
```
相关推荐
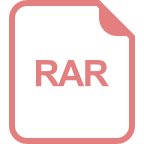
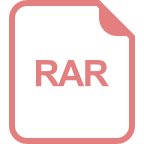
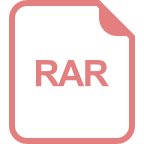















