请写出相应的python代码
时间: 2024-10-10 09:13:29 浏览: 21
当然,这里我会给你提供一个简单的Python版本的Ford-Fulkerson算法(最大流)和Dinic's Algorithm(也是求最大流的一种方法,适用于有向图中带权重的边)的例子。请注意,这仅是基础版,实际应用中可能会需要更复杂的库如networkx或Pandas。
**最大流 (Ford-Fulkerson) 示例:**
```python
from collections import defaultdict
def ford_fulkerson(graph, src, dest):
def augment_path(u, flow, graph, parent):
path_flow = 0
while u != dest:
path_flow += graph[u][parent][flow]
flow = min(flow, graph[parent][u])
u = parent
return path_flow
graph = {node: {} for node in graph}
max_flow = 0
residual_graph = {node: {} for node in graph}
for u, adjacencies in graph.items():
for v, capacity in adjacencies.items():
if v != src and v != dest:
residual_graph[u][v] = capacity
residual_graph[v][u] = 0
else:
residual_graph[u][v] = 0
residual_graph[v][u] = -capacity
while True:
prev = {src: None}
flow = dfs(residual_graph, src, dest, prev)
if flow == 0:
break
max_flow += flow
return max_flow
# 辅助函数:深度优先搜索(DFS)
def dfs(residual_graph, u, v, prev):
if u not in residual_graph or v not in residual_graph[u]:
return 0
if u == v:
return residual_graph[u][v]
path_flow = 0
for neighbor, flow in residual_graph[u].items():
if flow > 0 and prev.get(neighbor) is None:
new_flow = dfs(residual_graph, neighbor, v, {neighbor: u})
if new_flow > 0:
path_flow += new_flow
residual_graph[u][neighbor] -= new_flow
residual_graph[neighbor][u] += new_flow
return path_flow
# 使用示例:
graph = {
'A': {'B': 5, 'C': 3},
'B': {'A': 5, 'C': 2, 'D': 4},
'C': {'A': 3, 'B': 2, 'D': 1},
'D': {'B': 4, 'C': 1, 'E': 6},
'E': {'D': 6}
}
print(ford_fulkerson(graph, 'A', 'E')) # 输出最大流
```
**Dinic's Algorithm 示例(适用于带权边):**
```python
import heapq
def dinic(graph, src, sink):
INF = float('inf')
n = len(graph)
level = [None] * n
q = []
def bfs():
nonlocal q
level = [-1] * n
level[src] = 0
q.append(src)
while q:
curr = q.pop(0)
for nei, cap in graph[curr].items():
if level[nei] is None and cap > 0:
level[nei] = level[curr] + 1
q.append(nei)
flow = 0
while bfs():
push = []
for node in range(n):
if level[node] is None:
continue
for nei, cap in graph[node].items():
if cap > 0:
push.append((-cap, node, nei))
if not push:
break
flow += heapq.heappop(push)[0]
curr = sink
while curr != src:
graph[curr][level[curr]][curr], graph[level[curr]][curr][curr] = (
0,
graph[level[curr]][curr][curr] + graph[curr][level[curr]][curr],
)
curr = level[curr]
return flow
# 使用示例:
graph = {
'A': {'B': 5, 'C': 3},
'B': {'A': 5, 'C': 2, 'D': 4, 'E': 8},
'C': {'A': 3, 'B': 2, 'D': 1},
'D': {'B': 4, 'C': 1, 'E': 0},
'E': {'B': 8, 'D': 0, 'F': 3},
'F': {'E': 3}
}
print(dinic(graph, 'A', 'F')) # 输出最大流
```
阅读全文
相关推荐
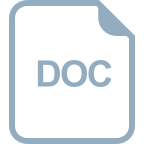
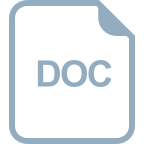
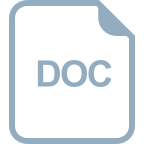















