keil怎么实现stm32的RS485
时间: 2024-05-28 14:09:47 浏览: 287
Keil可以通过使用HAL库中提供的函数来实现STM32的RS485通信,具体步骤如下:
1. 配置USART为RS485模式,可以通过修改USART的CR1寄存器来实现,将DE/RE引脚设置为输出模式,即可启用RS485模式。
2. 配置USART的收发缓冲区,通过使用HAL库提供的HAL_UART_Receive_IT()和HAL_UART_Transmit_IT()函数来实现,可以实现中断接收和发送。
3. 在发送完成后,需要控制DE/RE引脚的状态,切换为接收模式,以确保可以正确接收数据。可以通过GPIO库来设置引脚的状态。
4. 在接收完成后,需要将接收到的数据进行处理,可以自己定义处理函数来实现。
相关问题
keil5STM32F103使用RS485发送接受函数
以下是使用Keil5和STM32F103来进行RS485发送接收的函数示例:
```
#include "stm32f10x.h"
#define RS485_DIR_GPIO GPIOB
#define RS485_DIR_PIN GPIO_Pin_12
void RS485_SendData(uint8_t *pData, uint16_t len)
{
// Set RS485 Direction to Transmit
GPIO_WriteBit(RS485_DIR_GPIO, RS485_DIR_PIN, Bit_RESET);
// Send data using UART
for (uint16_t i = 0; i < len; i++)
{
USART_SendData(USART1, pData[i]);
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
}
// Wait for transmission complete
while (USART_GetFlagStatus(USART1, USART_FLAG_TC) == RESET);
// Set RS485 Direction to Receive
GPIO_WriteBit(RS485_DIR_GPIO, RS485_DIR_PIN, Bit_SET);
}
void RS485_ReceiveData(uint8_t *pData, uint16_t len)
{
// Set RS485 Direction to Receive
GPIO_WriteBit(RS485_DIR_GPIO, RS485_DIR_PIN, Bit_SET);
// Receive data using UART
for (uint16_t i = 0; i < len; i++)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
pData[i] = USART_ReceiveData(USART1);
}
}
```
在这个例子中,我们使用USART1来进行串口通信,同时使用一个GPIO引脚(PB12)来控制RS485芯片的发送/接收方向。在发送数据时,我们将RS485引脚设置为发送状态,然后使用USART1发送数据。在接收数据时,我们将RS485引脚设置为接收状态,然后使用USART1接收数据。需要注意的是,在接收数据时,为了防止丢失任何数据,我们必须等待USART_FLAG_RXNE标志位被设置为1,表示接收缓冲区中有可用的数据。
请注意,此代码仅用于参考,具体实现可能因应用程序而异。
keil5STM32F103使用RS485的中断函数
以下是基于Keil5的STM32F103使用RS485的中断函数的示例代码:
```c
#include "stm32f10x.h"
#define RS485_TX_ENABLE GPIO_SetBits(GPIOA, GPIO_Pin_1)
#define RS485_TX_DISABLE GPIO_ResetBits(GPIOA, GPIO_Pin_1)
uint8_t buffer[10];
uint8_t index = 0;
void RS485_Init(void)
{
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
USART_Cmd(USART1, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_1;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
RS485_TX_DISABLE;
}
void USART1_IRQHandler(void)
{
if(USART_GetITStatus(USART1, USART_IT_RXNE) != RESET)
{
buffer[index] = USART_ReceiveData(USART1);
index++;
}
}
void RS485_SendData(uint8_t* data, uint8_t len)
{
RS485_TX_ENABLE;
for(uint8_t i = 0; i < len; i++)
{
USART_SendData(USART1, data[i]);
while(USART_GetFlagStatus(USART1, USART_FLAG_TC) == RESET);
}
RS485_TX_DISABLE;
}
```
在这个例子中,我们通过USART1实现了RS485协议通信,并使用了USART的中断来接收数据。我们还定义了一个RS485_SendData函数,用于发送数据。在发送数据前,需要将RS485芯片的发送使能引脚置高,发送完成后,需要将其置低,以切换芯片的发送和接收模式。
需要注意的是,这只是一个简单的示例代码,具体的实现方式可能会因不同的硬件设计和具体应用场景而有所不同。
阅读全文
相关推荐
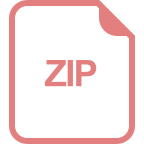


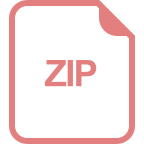
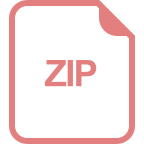
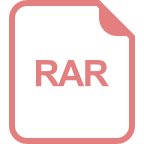
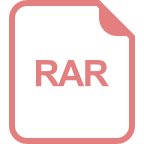
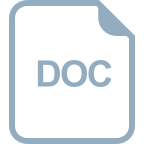
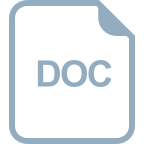




