import jqdata # 初始化聚宽账号密码 def initialize(context): # 设置回测日期区间 set_benchmark('000300.XSHG') set_option('use_real_price', True) # 设置买入的股票数量上限 g.max_stock_count = 5 def handle_data(context, data): # 获取当前日期 current_date = context.current_dt.date() # 获取股票池中的股票列表 stocks = get_index_stocks('000852.XSHG') # 按照股票池中的股票进行遍历 for stock in stocks: # 判断股票是否满足买入条件 if check_buy_condition(stock, current_date, context): buy_stock(stock, context) # 判断持有的股票是否满足卖出条件 if check_sell_condition(stock, current_date, context): sell_stock(stock, context) def check_buy_condition(stock, current_date, context): # 判断股票是否连续下跌三天 prices = attribute_history(stock,3, 'close', skip_paused=True) if len(prices) == 3 and prices['close'][-1] < prices['close'][-2] < prices['close'][-3]: return True else: return False def buy_stock(stock, context): # 判断当前持仓的股票数量是否已达上限 if len(context.portfolio.positions) >= g.max_stock_count: return # 买入股票 order_value(stock, context.portfolio.cash / g.max_stock_count) def check_sell_condition(stock, current_date, context): # 获取持有股票的买入日期 buy_date = context.portfolio.positions[stock].init_time.date() # 判断是否满足卖出条件 if current_date - buy_date >= 3: # 判断是否亏损超过5% if (context.portfolio.positions[stock].last_price - context.portfolio.positions[stock].avg_cost) / context.portfolio.positions[stock].avg_cost <= -0.05: return True return False def sell_stock(stock, context): # 卖出股票 order_target(stock, 0) 报错count = int(unit[:-1]) ValueError: invalid literal for int() with base 10: 'clos'
时间: 2024-04-09 10:30:52 浏览: 15
这个错误是因为在获取股票价格时,使用了错误的列名。在`attribute_history()`函数中,第三个参数应该是列名,而不是'close'。请将`attribute_history(stock,3, 'close', skip_paused=True)`修改为`attribute_history(stock, 3, 'close', skip_paused=True).close`。这样就可以正确获取股票的收盘价了。
相关问题
import jqdata # 初始化聚宽账号密码 def initialize(context): # 设置回测日期区间 set_benchmark('000300.XSHG') set_option('use_real_price', True) # 设置买入的股票数量上限 g.max_stock_count = 5 def handle_data(context, data): # 获取当前日期 current_date = context.current_dt.date() # 获取股票池中的股票列表 stocks = get_index_stocks('000852.XSHG') # 按照股票池中的股票进行遍历 for stock in stocks: # 判断股票是否满足买入条件 if check_buy_condition(stock, current_date, context): buy_stock(stock, context) # 判断持有的股票是否满足卖出条件 if check_sell_condition(stock, current_date, context): sell_stock(stock, context) def check_buy_condition(stock, current_date, context): # 判断股票是否连续下跌三天 prices = attribute_history(stock, 3, '1d', ['close']) if len(prices) == 3 and prices['close'][-1] < prices['close'][-2] < prices['close'][-3]: return True else: return False def buy_stock(stock, context): # 判断当前持仓的股票数量是否已达上限 if len(context.portfolio.positions) >= g.max_stock_count: return # 买入股票 order_value(stock, context.portfolio.cash / g.max_stock_count) def check_sell_condition(stock, current_date, context): # 获取持有股票的买入日期 buy_date = context.portfolio.positions[stock].init_time.date() # 判断是否满足卖出条件 if current_date - buy_date >= 3: # 判断是否亏损超过5% if (context.portfolio.positions[stock].last_price - context.portfolio.positions[stock].avg_cost) / context.portfolio.positions[stock].avg_cost <= -0.05: return True return False def sell_stock(stock, context): # 卖出股票 order_target(stock, 0)当中buy_date = context.portfolio.positions[stock].init_time.date()报错'NoneType' object has no attribute 'date'
这个错误是因为在`check_sell_condition`函数中,`context.portfolio.positions[stock].init_time`返回了`None`,没有日期属性。这可能是因为你在买入股票之前没有设置买入日期。你可以在买入股票时设置买入日期,例如:
```python
def buy_stock(stock, context):
# 判断当前持仓的股票数量是否已达上限
if len(context.portfolio.positions) >= g.max_stock_count:
return
# 设置买入日期
buy_date = context.current_dt.date()
# 买入股票
order_value(stock, context.portfolio.cash / g.max_stock_count, date=buy_date)
```
然后在`check_sell_condition`函数中,你可以直接使用`buy_date`判断是否满足卖出条件:
```python
def check_sell_condition(stock, current_date, context):
# 获取持有股票的买入日期
buy_date = context.portfolio.positions[stock].init_time.date()
# 判断是否满足卖出条件
if current_date - buy_date >= 3:
# 判断是否亏损超过5%
if (context.portfolio.positions[stock].last_price - context.portfolio.positions[stock].avg_cost) / context.portfolio.positions[stock].avg_cost <= -0.05:
return True
return False
```
这样就可以避免报错了。
将股票均线策略转换为聚宽代码
聚宽是国内一家量化投资平台,提供了股票、期货、基金等市场的历史数据、实时行情和量化交易等服务。下面是将股票均线策略转换为聚宽代码的示例:
```python
# 导入聚宽数据包
import jqdata
# 初始化函数,设定要操作的股票、基准等等
def initialize(context):
# 设定要操作的股票:平安银行(000001.XSHE)
g.security = '000001.XSHE'
# 设定均线时间窗口为20日
g.days = 20
# 设定基准收益:沪深300指数
set_benchmark('000300.XSHG')
# 定义股票均线策略
def handle_data(context, data):
# 获取股票历史价格数据
prices = history(g.days, '1d', 'close')[g.security]
# 计算股票的均线
ma = prices.mean()
# 判断当前市场趋势
if prices[-1] > ma:
# 当前价格高于均线,买入股票
order_target_value(g.security, context.portfolio.total_value)
else:
# 当前价格低于均线,卖出股票
order_target_value(g.security, 0)
```
以上代码实现了简单的股票均线策略,当股票收盘价高于20日均线时,买入该股票;当股票收盘价低于20日均线时,卖出该股票。该策略可以在聚宽平台上进行回测和实盘交易。
相关推荐
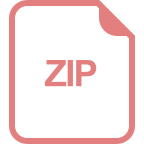
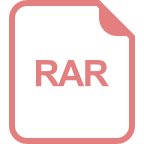








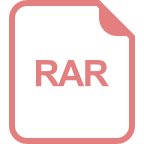
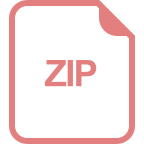