C++类中的has-a关系和is-a关系
时间: 2023-12-18 07:03:22 浏览: 73
has-a关系是指类中包含了其他类的对象作为自己的成员变量,表示一个类拥有另外一个类的实例。比如一个汽车类可以包含一个引擎类的对象作为自己的成员变量,表示汽车拥有一个引擎。
is-a关系是指类之间的继承关系,表示一个类是另外一个类的一种特殊形式。比如一个圆形类可以继承自一个图形类,表示圆形是一种图形。在C++中,使用关键字“extends”来表示继承关系。
相关问题
sudo ./bootstrap --------------------------------------------- CMake 3.8.1, Copyright 2000-2017 Kitware, Inc. and Contributors Found GNU toolchain C compiler on this system is: gcc C++ compiler on this system is: g++ Makefile processor on this system is: make g++ is GNU compiler g++ has setenv g++ has unsetenv g++ does not have environ in stdlib.h g++ has stl wstring g++ has <ext/stdio_filebuf.h> --------------------------------------------- make: 'cmake' is up to date. CMake Error: The current CMakeCache.txt directory /home/GPU_Project/ARM-Mali-G72/Software_packet/DDK-Compile_Tool/cmake/cmake-3.8.1/CMakeCache.txt is different than the directory /home/test/GPU_Project/ARM-Mali-G72/Software_packet/DDK-Compile_Tool/cmake/cmake-3.8.1 where CMakeCache.txt was created. This may result in binaries being created in the wrong place. If you are not sure, reedit the CMakeCache.txt --------------------------------------------- Error when bootstrapping CMake: Problem while running initial CMake ---------------------------------------------
这个错误信息提示是在运行 CMake 过程中出现了问题,可能是由于 CMakeCache.txt 文件所在的目录不一致导致的。建议检查一下 CMakeCache.txt 文件所在的目录是否正确,并且尝试清空 CMake 缓存重新运行 bootstrap。你可以尝试以下命令:
```
rm -rf CMakeCache.txt
sudo ./bootstrap
```
如果还有问题,可以提供更多的错误信息,以便更好地帮助你解决问题。
Given a pair of positive integers, for example, 6 and 110, can this equation 6 = 110 be true? The answer is yes, if 6 is a decimal number and 110 is a binary number. Now for any pair of positive integers N1 and N2, your task is to find the radix of one number while that of the other is given. Input Specification: Each input file contains one test case. Each case occupies a line which contains 4 positive integers: N1 N2 tag radix Here N1 and N2 each has no more than 10 digits. A digit is less than its radix and is chosen from the set { 0-9, a-z } where 0-9 represent the decimal numbers 0-9, and a-z represent the decimal numbers 10-35. The last number radix is the radix of N1 if tag is 1, or of N2 if tag is 2. Output Specification: For each test case, print in one line the radix of the other number so that the equation N1 = N2 is true. If the equation is impossible, print Impossible. If the solution is not unique, output the smallest possible radix. 用c++写代码 并且要考虑radix大于36的情况
您想解决一个关于进制转换的问题,其中需要确定一个数字的进制,而另一个数字的进制已知。您希望用C++编写代码,并且要考虑进制大于36的情况。以下是一个可能的实现:
```cpp
#include <iostream>
#include <string>
#include <cmath>
using namespace std;
int charToInt(char c) {
if (isdigit(c)) {
return c - '0';
} else {
return c - 'a' + 10;
}
}
long long convertToDecimal(string num, int radix) {
long long result = 0;
int power = 0;
for (int i = num.length() - 1; i >= 0; i--) {
result += charToInt(num[i]) * pow(radix, power);
power++;
}
return result;
}
int findRadix(string num, long long target) {
char maxDigit = *max_element(num.begin(), num.end());
int minRadix = charToInt(maxDigit) + 1; // Smallest possible radix
// Binary search to find the radix
int left = minRadix;
int right = max(36, minRadix); // Consider radix larger than 36
while (left <= right) {
int mid = (left + right) / 2;
long long converted = convertToDecimal(num, mid);
if (converted == target) {
return mid;
} else if (converted < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Impossible
}
int main() {
string N1, N2;
int tag, radix;
cin >> N1 >> N2 >> tag >> radix;
if (tag == 1) {
long long decimalN2 = convertToDecimal(N2, radix);
int resultRadix = findRadix(N1, decimalN2);
if (resultRadix == -1) {
cout << "Impossible" << endl;
} else {
cout << resultRadix << endl;
}
} else {
long long decimalN1 = convertToDecimal(N1, radix);
int resultRadix = findRadix(N2, decimalN1);
if (resultRadix == -1) {
cout << "Impossible" << endl;
} else {
cout << resultRadix << endl;
}
}
return 0;
}
```
这个程序首先定义了一个`charToInt`函数,用于将字符转换为对应的整数值。然后,`convertToDecimal`函数将给定进制的数字转换为十进制数。最后,`findRadix`函数使用二分查找来确定另一个数字的进制。在主函数中,根据输入的`tag`值选择相应的计算方法,并输出结果。
希望这可以帮助到您!如果您有任何其他问题,请随时问我。
阅读全文
相关推荐
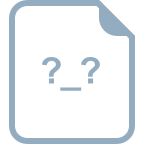
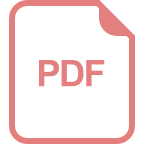
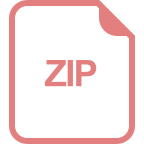











