协调器可以通过串口控制终端或路由器的灯亮和灭。可以自定义通信协 议,比如:第 0 个字节为‘l’表示控制灯消息,第 1 个字节为设备 ID,第 2 个字节为灯的编号(1 或 2),第 3 字节为灯的灯的亮灭(0 灭,1 亮)C语言代码
时间: 2024-02-28 16:55:35 浏览: 73
以下是一个简单的C语言代码示例,用于实现协调器通过串口控制设备灯亮和灭的功能。假设协调器可以向终端或路由器发送控制灯的消息,并通过自定义通信协议指定设备ID、灯的编号和灯的状态(亮或灭)。
```c
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
// 定义串口设备路径
#define SERIAL_DEVICE "/dev/ttyS0"
// 定义控制灯的消息类型
#define CONTROL_LED_MSG_TYPE 'l'
// 定义串口通信协议结构体
struct SerialProtocol {
char type; // 消息类型
char deviceId; // 设备ID
char led; // 灯的编号(1或2)
char status; // 灯的状态(0灭,1亮)
};
// 打开串口设备
int openSerialDevice() {
int fd = open(SERIAL_DEVICE, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("Open serial device failed");
return -1;
}
// 配置串口参数
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cc[VTIME] = 0;
options.c_cc[VMIN] = 1;
tcsetattr(fd, TCSANOW, &options);
return fd;
}
// 发送控制灯的消息
int sendControlLedMessage(int fd, char deviceId, char led, char status) {
// 构造串口通信协议结构体
struct SerialProtocol protocol;
protocol.type = CONTROL_LED_MSG_TYPE;
protocol.deviceId = deviceId;
protocol.led = led;
protocol.status = status;
// 发送消息
int n = write(fd, &protocol, sizeof(protocol));
if (n < 0) {
perror("Write serial device failed");
return -1;
}
return 0;
}
int main() {
// 打开串口设备
int fd = openSerialDevice();
if (fd < 0) {
return -1;
}
// 发送控制灯的消息
char deviceId = 1;
char led = 2;
char status = 1;
sendControlLedMessage(fd, deviceId, led, status);
// 关闭串口设备
close(fd);
return 0;
}
```
在上面的例子中,我们定义了一个串口通信协议结构体,包含了控制灯的消息类型、设备ID、灯的编号和灯的状态。打开串口设备时,我们先配置了串口参数。发送控制灯的消息时,我们先构造了串口通信协议结构体,然后将结构体写入串口设备。最后,我们关闭了串口设备。
阅读全文
相关推荐
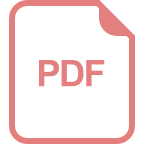
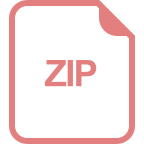
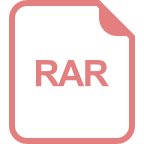
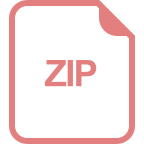
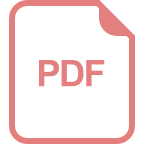
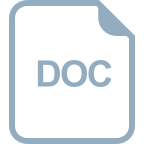
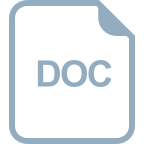
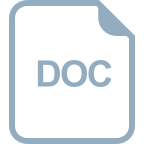
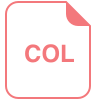
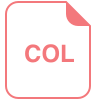
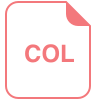
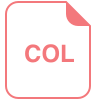
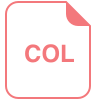
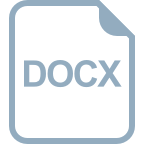