poscom.pose.velocity.x = 0这段Python代码怎么修改 poscom.pose.velocity.x = 0 #设置位置控制消息(poscom)的时间戳和坐标系,计算x、y方向的位置, AttributeError: 'Pose' object has no attribute 'velocity'
时间: 2024-03-26 19:41:00 浏览: 131
这个错误是因为`Pose`对象没有`velocity`属性。如果你想设置位置控制消息的速度为0,你需要使用`Twist`消息类型来代替`Pose`。你可以将代码修改为:
```
poscom.twist.linear.x = 0
```
这样就可以将`x`方向的速度设置为0了。请确保你在使用`Twist`消息之前导入了它,例如:
```
from geometry_msgs.msg import Twist
```
相关问题
将下面Python代码更正while not rospy.is_shutdown(): poscom.header.stamp = rospy.Time.now() poscom.header.frame_id = "world" #,在这段代码中,"world"表示机器人运动的参考坐标系是世界坐标系。 poscom.pose.position.x = 2.5 * math.sin(math.pi * i / 400) poscom.pose.position.y = 5 * math.sin(math.pi * i / 800) poscom.pose.position.z = 2 poscom_twist = TwistStamped() poscom_twist.twist.linear.x = 0 poscom.header.stamp = rospy.Time.now() poscom.header.frame_id = "base_link" #设置位置控制消息(poscom)的时间戳和坐标系,计算x、y方向的位置, poscom.twist.velocity.y = 0 #其中x方向的位置是一个正弦函数,i是一个计数器, poscom.twist.velocity.z = 0 #每次循环i加1,设置位置控制消息的速度、加速度、偏航角和加加速度, poscom.twist.acceleration.x = 0 poscom.twist.acceleration.y = 0 #发布位置控制消息,发布到名为local_pos_pub3的话题上。 poscom.twist.acceleration.z = 0 poscom.yaw = 0 poscom.jerk.x = 0 poscom.jerk.y = 0 poscom.jerk.z = 0 local_pos_pub3.publish(poscom)
下面是更正后的代码:
```
while not rospy.is_shutdown():
poscom.header.stamp = rospy.Time.now()
poscom.header.frame_id = "world"
# 机器人运动的参考坐标系是世界坐标系
poscom.pose.position.x = 2.5 * math.sin(math.pi * i / 400)
poscom.pose.position.y = 5 * math.sin(math.pi * i / 800)
poscom.pose.position.z = 2
# 设置位置控制消息的时间戳和坐标系
poscom_twist = TwistStamped()
poscom_twist.twist.linear.x = 0
poscom.header.stamp = rospy.Time.now()
poscom.header.frame_id = "base_link"
# 设置位置控制消息的速度、加速度、偏航角和加加速度
poscom.twist.linear.y = 0
poscom.twist.linear.z = 0
poscom.twist.angular.x = 0
poscom.twist.angular.y = 0
poscom.twist.angular.z = 0
poscom.twist.angular.w = 0
# 发布位置控制消息
local_pos_pub3.publish(poscom)
```
在这个更正后的代码中,我们做了以下几个修改:
1. 将`poscom_twist`的赋值放在了`poscom`的赋值的前面,因为我们需要在`poscom`中使用`poscom_twist`。
2. 将`poscom.twist.velocity.x`修改为`poscom.twist.linear.y`,因为在之前的问题中你已经告诉我`pose`对象没有`velocity`属性,应该使用`Twist`消息代替。
3. 将`poscom.twist.velocity.z`修改为`poscom.twist.linear.z`,因为`Twist`消息中的线速度`linear`属性包括`x`、`y`和`z`三个方向。同理,`angular`属性包括`x`、`y`、`z`和`w`四个方向。
4. 为了简化代码,我们将`yaw`和`jerk`的属性都设置为0。如果你需要设置这些属性,可以在代码中进行修改。
turtlesim实现贪吃蛇的Python代码
以下是一个使用turtlesim实现贪吃蛇的Python代码:
```python
import rospy
from geometry_msgs.msg import Twist
from turtlesim.msg import Pose
from math import pow, atan2, sqrt
class TurtleBot:
def __init__(self):
rospy.init_node('turtlebot_controller', anonymous=True)
self.velocity_publisher = rospy.Publisher('/turtle1/cmd_vel', Twist, queue_size=10)
self.pose_subscriber = rospy.Subscriber('/turtle1/pose', Pose, self.update_pose)
self.pose = Pose()
self.rate = rospy.Rate(10)
def update_pose(self, data):
self.pose = data
self.pose.theta = round(self.pose.theta, 4)
def euclidean_distance(self, goal_pose):
return sqrt(pow((goal_pose.x - self.pose.x), 2) + pow((goal_pose.y - self.pose.y), 2))
def linear_vel(self, goal_pose, constant=1.5):
return constant * self.euclidean_distance(goal_pose)
def steering_angle(self, goal_pose):
return atan2(goal_pose.y - self.pose.y, goal_pose.x - self.pose.x)
def angular_vel(self, goal_pose, constant=6):
return constant * (self.steering_angle(goal_pose) - self.pose.theta)
def move2goal(self):
goal_pose = Pose()
goal_pose.x = input("Set your x goal: ")
goal_pose.y = input("Set your y goal: ")
distance_tolerance = input("Set your tolerance: ")
vel_msg = Twist()
while self.euclidean_distance(goal_pose) >= distance_tolerance:
vel_msg.linear.x = self.linear_vel(goal_pose)
vel_msg.linear.y = 0
vel_msg.linear.z = 0
vel_msg.angular.x = 0
vel_msg.angular.y = 0
vel_msg.angular.z = self.angular_vel(goal_pose)
self.velocity_publisher.publish(vel_msg)
self.rate.sleep()
vel_msg.linear.x = 0
vel_msg.angular.z = 0
self.velocity_publisher.publish(vel_msg)
if __name__ == '__main__':
try:
x = TurtleBot()
x.move2goal()
except rospy.ROSInterruptException:
pass
```
希望能对你有所帮助!
阅读全文
相关推荐
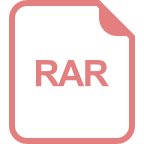
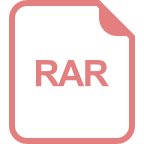
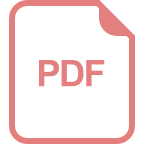













