在ROS下编写代码,利用编写的代码来控制小乌龟的运动,包括但不限于直线运动、圆周运动、转向,速度变化,并录制数据包。
时间: 2023-10-10 15:12:53 浏览: 347
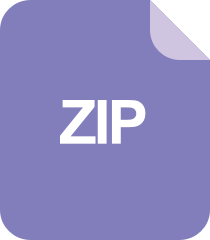
ROS机械臂运动控制(完整项目代码python)

好的,这个任务需要先安装ROS,并且需要安装Turtlesim模拟器和rosbag包。
安装Turtlesim模拟器:
```bash
sudo apt-get install ros-melodic-turtlesim
```
安装rosbag包:
```bash
sudo apt-get install ros-melodic-rosbag
```
接下来,我们可以在ROS中创建一个新的工作空间:
```bash
mkdir -p ~/turtlebot_ws/src
cd ~/turtlebot_ws/src
catkin_init_workspace
```
然后,我们需要创建一个ROS包来管理我们的代码:
```bash
cd ~/turtlebot_ws/src
catkin_create_pkg turtlebot_control rospy roscpp turtlesim
```
在src文件夹中创建一个名为turtlebot_control.py的Python文件,并将下面的代码复制到文件中:
```python
#!/usr/bin/env python
import rospy
from geometry_msgs.msg import Twist
from turtlesim.msg import Pose
from math import pow, atan2, sqrt
class TurtleBot:
def __init__(self):
# 初始化节点
rospy.init_node('turtlebot_control', anonymous=True)
# 发布器和订阅器
self.velocity_publisher = rospy.Publisher('/turtle1/cmd_vel', Twist, queue_size=10)
self.pose_subscriber = rospy.Subscriber('/turtle1/pose', Pose, self.update_pose)
self.pose = Pose()
self.rate = rospy.Rate(10)
# 回调函数,更新小乌龟的位置和朝向
def update_pose(self, data):
self.pose = data
self.pose.x = round(self.pose.x, 4)
self.pose.y = round(self.pose.y, 4)
# 计算小乌龟和目标点之间的距离
def euclidean_distance(self, goal_pose):
return sqrt(pow((goal_pose.x - self.pose.x), 2) + pow((goal_pose.y - self.pose.y), 2))
# 计算小乌龟和目标点之间的角度
def linear_vel(self, goal_pose, constant=1.5):
return constant * self.euclidean_distance(goal_pose)
# 计算小乌龟需要旋转的角度
def steering_angle(self, goal_pose):
return atan2(goal_pose.y - self.pose.y, goal_pose.x - self.pose.x)
# 计算小乌龟需要旋转的方向
def angular_vel(self, goal_pose, constant=6):
return constant * (self.steering_angle(goal_pose) - self.pose.theta)
# 小乌龟在直线上移动的函数
def move2goal(self):
goal_pose = Pose()
goal_pose.x = input("Set your x goal: ")
goal_pose.y = input("Set your y goal: ")
distance_tolerance = 0.1
vel_msg = Twist()
while self.euclidean_distance(goal_pose) >= distance_tolerance:
# 线性速度
vel_msg.linear.x = self.linear_vel(goal_pose)
vel_msg.linear.y = 0
vel_msg.linear.z = 0
# 角速度
vel_msg.angular.x = 0
vel_msg.angular.y = 0
vel_msg.angular.z = self.angular_vel(goal_pose)
# 发布速度消息
self.velocity_publisher.publish(vel_msg)
# 延迟,使循环以10Hz的速度运行
self.rate.sleep()
# 停止小乌龟运动
vel_msg.linear.x = 0
vel_msg.angular.z = 0
self.velocity_publisher.publish(vel_msg)
# 小乌龟在圆周上运动的函数
def circle(self):
radius = input("Set your radius: ")
vel_msg = Twist()
while not rospy.is_shutdown():
# 线速度
vel_msg.linear.x = 2 * 3.14 * radius / 10
vel_msg.linear.y = 0
vel_msg.linear.z = 0
# 角速度
vel_msg.angular.x = 0
vel_msg.angular.y = 0
vel_msg.angular.z = 2 * 3.14 / 20
# 发布速度消息
self.velocity_publisher.publish(vel_msg)
# 延迟,使循环以10Hz的速度运行
self.rate.sleep()
# 停止小乌龟运动
vel_msg.linear.x = 0
vel_msg.angular.z = 0
self.velocity_publisher.publish(vel_msg)
# 小乌龟转向的函数
def turn(self):
angle = input("Set your angle: ")
vel_msg = Twist()
# 角速度为正,小乌龟向左转
vel_msg.angular.z = 0.5
# 弧度与角度的转换
radians = angle * 3.14 / 180
# 计算小乌龟需要旋转的时间
t0 = rospy.Time.now().to_sec()
current_angle = 0
while current_angle < radians:
self.velocity_publisher.publish(vel_msg)
t1 = rospy.Time.now().to_sec()
current_angle = 0.5 * (t1 - t0)
# 停止小乌龟运动
vel_msg.angular.z = 0
self.velocity_publisher.publish(vel_msg)
# 录制数据包的函数
def record(self):
filename = input("Please enter the filename: ")
duration = input("Please enter the duration of recording: ")
# 创建rosbag记录器
command = 'rosbag record -O ' + filename + ' /turtle1/cmd_vel /turtle1/pose -q -a --duration=' + str(duration)
os.system(command)
if __name__ == '__main__':
try:
turtlebot = TurtleBot()
# 移动小乌龟
print("Move to goal")
turtlebot.move2goal()
# 在圆周上运动
print("Circle")
turtlebot.circle()
# 转向
print("Turn")
turtlebot.turn()
# 录制数据包
print("Record")
turtlebot.record()
rospy.spin()
except rospy.ROSInterruptException:
pass
```
这个代码中包含了一些函数来控制小乌龟的运动,包括直线运动、圆周运动、转向和录制数据包。你可以根据需要进行修改,并在终端中使用下面的命令来运行代码:
```bash
cd ~/turtlebot_ws
catkin_make
source devel/setup.bash
rosrun turtlebot_control turtlebot_control.py
```
如果需要查看数据包,可以在终端中使用下面的命令:
```bash
cd ~/turtlebot_ws/src/turtlebot_control
rosbag play <filename>
```
阅读全文
相关推荐
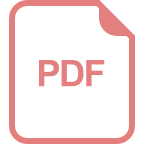
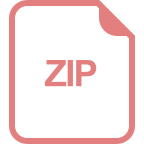















