stm32单片机按键防抖代码
时间: 2024-05-02 18:15:03 浏览: 161
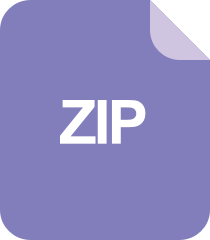
7、stm32单片机之模拟按键控制单个舵机.zip
STM32单片机按键防抖是为了解决按键在按下或释放时可能会产生的抖动现象,使得按键信号更加稳定可靠。以下是一种常见的STM32单片机按键防抖代码实现方式:
```c
#include "stm32f10x.h"
#define KEY_GPIO_PORT GPIOA
#define KEY_GPIO_PIN GPIO_Pin_0
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = KEY_GPIO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(KEY_GPIO_PORT, &GPIO_InitStructure);
}
uint8_t Key_Debounce(void)
{
static uint8_t key_state = 0; // 按键状态,0表示未按下,1表示按下
static uint8_t key_cnt = 0; // 按键计数器,用于消抖
if (GPIO_ReadInputDataBit(KEY_GPIO_PORT, KEY_GPIO_PIN) == 0) // 检测按键是否按下
{
if (key_cnt < 10) // 消抖计数器小于10时,继续计数
{
key_cnt++;
}
else if (key_cnt == 10) // 消抖计数器等于10时,表示按键已经稳定按下
{
key_state = 1;
key_cnt++;
}
}
else // 按键未按下
{
if (key_cnt > 0) // 消抖计数器大于0时,继续计数
{
key_cnt--;
}
else if (key_cnt == 0) // 消抖计数器等于0时,表示按键已经稳定释放
{
key_state = 0;
}
}
return key_state;
}
int main(void)
{
GPIO_Configuration();
while (1)
{
if (Key_Debounce() == 1) // 检测按键是否按下
{
// 执行按键按下后的操作
}
}
}
```
以上代码中,`GPIO_Configuration`函数用于配置按键所在的GPIO引脚为输入模式,并开启相应的时钟。`Key_Debounce`函数用于实现按键的防抖功能,通过计数器判断按键是否稳定按下或释放,并返回相应的状态。在主函数中,通过调用`Key_Debounce`函数来检测按键是否按下,并执行相应的操作。
阅读全文
相关推荐
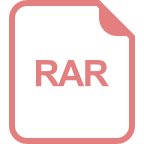
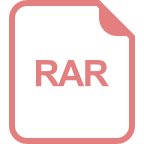















