完善point_to_polygon_distance
时间: 2024-10-04 11:03:29 浏览: 29
完善`point_to_polygon_distance`函数的具体实现会涉及到一些几何操作和数据结构。这里是一个简化的示例,使用了射线投射法的基本思路,并假设我们已经有了一个包含点和多边形顶点的数据结构:
```cpp
#include <CGAL/Kd_tree.h>
#include <CGAL/Segment_d_traits_2.h>
#include <CGAL/Segment_Distance_2.h>
// 定义点和多边形
using namespace CGAL;
struct Point {
double x, y;
};
class Polygon {
public:
std::vector<Point> vertices; // 多边形顶点集合
typedef Kd_tree<Segment_dTraits_2> KdTreeType;
KdTreeType kd_tree; // 利用K-d树加速查找
};
// 创建CGAL Segment_Distance_2实例
typedef Segment_Distance_2<KdTreeType::FT> Distance;
double point_to_polygon_distance(const Point& p, const Polygon& poly) {
Distance distance_calculator;
// 创建射线,从p开始向负y方向无限延伸
Segment_d s(p.x, p.y, -std::numeric_limits<double>::infinity(), -std::numeric_limits<double>::infinity());
// 用于存储最近的交点和其距离
Point nearest_intersection;
double min_distance = std::numeric_limits<double>::max();
// 使用K-d树搜索最接近点p的多边形边界
for (const auto& vertex : poly.vertices) {
if (!distance_calculator.distance(s, Segment_d(vertex.x, vertex.y, vertex.x, vertex.y), nearest_intersection, min_distance)) {
break;
}
}
// 如果找到交点并且比原点离得多,返回交点到原点的距离;否则返回无穷大
return min_distance > 0 ? min_distance : std::numeric_limits<double>::infinity();
}
int main() {
Point p(0, 0);
Polygon poly; // 填充多边形顶点
double dist = point_to_polygon_distance(p, poly);
return 0;
}
```
注意,这个例子依赖于`CGAL`库,它提供了一些高效的几何计算工具,如K-d树和点到线段的快速距离计算。如果你不打算使用第三方库,那么你需要自行实现点到线段的距离计算以及射线与多边形边界的相交判断。
阅读全文
相关推荐
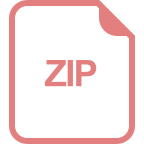
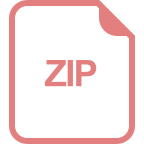
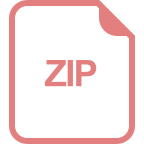















