创建两个单链表LA=(1,2.5),LB=(2,4,6,》,编写算法,将它们 合并成一个单链表LC,要求LC也是非递减有序排列
时间: 2024-12-22 12:28:39 浏览: 6
要将两个已排序的单链表 LA 和 LB 合并成一个新的非递减有序链表 LC,你可以采用双指针法,同时遍历两个链表,每次选择较小的元素添加到新链表中。如果遇到一个链表的当前节点比另一个大,则继续遍历那个链表直到找到更小的节点。以下是一个 C++ 实现的例子:
```cpp
#include <iostream>
// 定义链表节点结构体
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
};
// 合并两个链表
ListNode* mergeSortedLists(ListNode* la, ListNode* lb) {
// 创建新链表头节点
ListNode* lc = nullptr;
ListNode* tail = nullptr;
// 指针遍历
while (la != nullptr && lb != nullptr) {
if (la->val <= lb->val) {
// 如果LA节点值较小,添加到LC,并更新指针
if (lc == nullptr) {
lc = la;
tail = la;
} else {
tail->next = la;
tail = tail->next;
}
la = la->next;
} else {
// 否则,LB节点值较小,同样处理
if (lc == nullptr) {
lc = lb;
tail = lb;
} else {
tail->next = lb;
tail = tail->next;
}
lb = lb->next;
}
}
// 将剩余链表尾部连接到LC(如果存在)
if (la != nullptr) {
tail->next = la;
} else if (lb != nullptr) {
tail->next = lb;
}
return lc;
}
// 打印链表
void printList(ListNode* head) {
ListNode* temp = head;
while (temp != nullptr) {
std::cout << temp->val << " ";
temp = temp->next;
}
std::cout << std::endl;
}
int main() {
// 假设LA 和 LB 是已知的链表
ListNode* la = new ListNode(1);
la->next = new ListNode(2.5);
ListNode* lb = new ListNode(2);
lb->next = new ListNode(4);
lb->next->next = new ListNode(6);
// 合并链表
ListNode* lc = mergeSortedLists(la, lb);
// 输出结果
printList(lc);
// 清理内存
delete la;
delete lb;
delete lc; // 如果lc不是临时变量,记得删除
return 0;
}
```
运行这个程序后,将会输出合并后的链表 `LC` 的值:`1 2 2.5 4 6`。
阅读全文
相关推荐
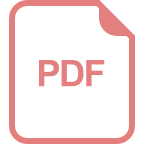
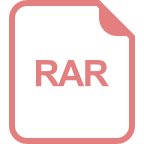
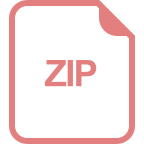


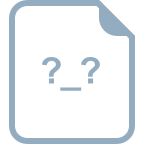
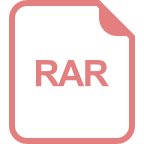
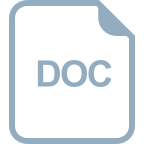
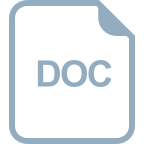










