请写出这道题的c++代码
时间: 2024-10-02 21:01:31 浏览: 32
为了编写C++代码来解决这个问题,我们可以创建一个`HistoryRecord`类,用于存储网址及其索引,同时实现一个队列(先进先出)来模拟历史记录的行为。下面是可能的一个解决方案:
```cpp
#include <iostream>
#include <queue>
#include <vector>
class HistoryRecord {
public:
int index;
std::string url;
HistoryRecord(int i, const std::string& u)
: index(i), url(u) {}
};
std::string processCommand(const std::string& command, int capacity, std::queue<HistoryRecord>& history) {
std::istringstream iss(command);
int op, num;
if (!(iss >> op >> num)) {
return "Invalid input";
}
switch (op) {
case 1: // 打开新网址
if (history.size() == capacity) {
history.pop();
}
history.push(HistoryRecord(history.size() + 1, "New URL " + std::to_string(history.size() + 1)));
break;
case 2: // 前进
if (num <= history.size()) {
if (num == 1) {
return std::to_string(history.front().index);
} else {
for (int i = 1; i < num; ++i) {
history.pop();
}
return std::to_string(history.front().index);
}
} else {
return "Error: no forward history";
}
case 3: // 后退
if (num > 0) {
if (num <= history.size()) {
return std::to_string(history[history.size() - num].index);
} else {
return "Error: no back history";
}
} else {
return "Invalid input";
}
case 4: // 退出
history.clear();
return "";
default:
return "Invalid operation";
}
}
int main() {
int capacity;
std::cin >> capacity;
std::string command;
while (std::getline(std::cin, command), command != "0") {
auto result = processCommand(command, capacity, history_queue);
if (!result.empty()) {
std::cout << result << "\n";
}
}
return 0;
}
```
在这个代码中,我们首先读取用户的输入,然后通过`processCommand`函数解析每个操作,根据操作类型执行相应的逻辑。主要的数据结构是`std::queue<HistoryRecord>`,它提供了自然的前进和后退功能。记得在实际运行前初始化`history_queue`变量。
阅读全文
相关推荐
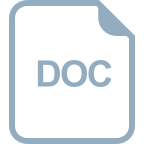
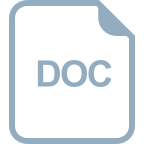
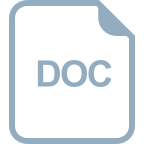
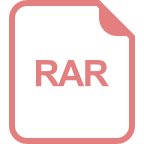














