c语言,集合A和集合B,生成集合C=A∩B算法
时间: 2024-10-09 10:14:09 浏览: 70
在C语言中,生成集合C,即集合A和集合B的交集(A∩B),通常涉及两个步骤:遍历和比较元素。如果元素同时存在于A和B中,就将其添加到集合C。这里可以使用数组、链表或者哈希表等数据结构来存储集合。
以下是使用数组的一个简单示例,假设我们有两组整数作为集合:
```c
#include <stdio.h>
#include <stdbool.h>
// 定义集合的数据结构
typedef struct {
int *elements; // 集合元素的指针
int count; // 集合元素的数量
} Set;
// 添加元素到集合
void add_element(Set *set, int element) {
set->elements = realloc(set->elements, (set->count + 1) * sizeof(int));
set->elements[set->count++] = element;
}
// 检查元素是否在集合中
bool is_in_set(const Set *set, int element) {
for (int i = 0; i < set->count; ++i) {
if (set->elements[i] == element) return true;
}
return false;
}
// 生成交集
Set* intersection(Set *a, Set *b) {
Set *result = malloc(sizeof(Set));
result->elements = NULL;
result->count = 0;
for (int i = 0; i < a->count; ++i) {
if (is_in_set(b, a->elements[i])) {
add_element(result, a->elements[i]);
}
}
return result;
}
// 示例
int main() {
Set A = { .elements = {1, 2, 3, 4, 5}, .count = 5 };
Set B = { .elements = {3, 4, 5, 6, 7}, .count = 5 };
Set *intersection_set = intersection(&A, &B);
// 输出交集
printf("Intersection: ");
for (int i = 0; i < intersection_set->count; ++i) {
printf("%d ", intersection_set->elements[i]);
}
free(intersection_set);
return 0;
}
```
这个例子中,`intersection`函数会创建一个新的集合C,包含A和B共同的元素。请注意,实际项目中可能会选择更高效的数据结构,如哈希表,以提高查找效率。
阅读全文
相关推荐
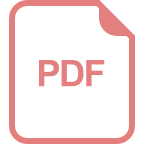

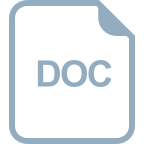
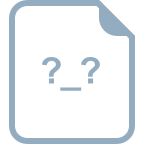
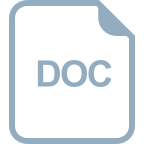
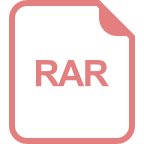
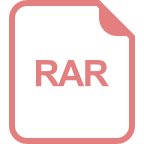
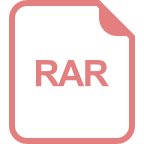
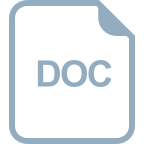
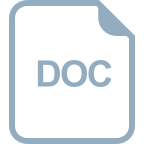
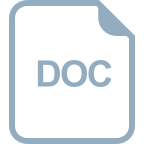
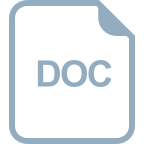
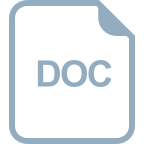
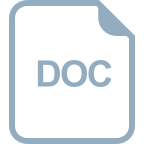




