用python语言实现对多边形Delaunay三角剖分,需使用Lawson算法。详细注释
时间: 2024-12-13 16:14:52 浏览: 16
在Python中,我们可以利用`scipy.spatial`库中的`voronoi`函数结合自定义的Lawson算法来实现多边形的Delaunay三角剖分。Lawson算法是一种用于快速构建Delaunay三角网的迭代改进算法。以下是一个简单的示例,展示了如何使用`scipy`和自定义的Lawson算法:
```python
from scipy.spatial import Voronoi, voronoi_plot_2d
import numpy as np
# 定义一个多边形
def polygon(n_sides):
angles = np.linspace(0, 2*np.pi, n_sides + 1)
return [(np.cos(a), np.sin(a)) for a in angles[:-1]]
# 自定义Lawson算法实现Delaunay三角化
def delaunay_lawson(points):
if len(points) <= 3:
return Voronoi(points)
# 初始化Voronoi图
vor = Voronoi(points)
# 获取最远点
farthest_point_index = np.argmax(np.linalg.norm(vor.points - points[0], axis=1))
# 遍历所有点,删除最远离已知点的点
while True:
farthest_point = vor.vertices[farthest_point_index]
vor.delete_vertices([farthest_point_index])
# 使用Lawson准则判断新形成的面是否违反Delaunay条件
for neighbor in vor.regions[vor.point_region[farthest_point_index]]:
if neighbor != -1 and np.any(np.linalg.norm(farthest_point - vor.vertices[neighbor], axis=1) > np.linalg.norm(farthest_point - vor.points[0])):
break
else:
# 如果没有违反,添加新的点并跳出循环
vor.vertices.append(farthest_point)
vor.regions.append(-1)
break
# 更新最远离已知点的点
farthest_point_index = np.argmax(np.linalg.norm(vor.points - farthest_point, axis=1))
return vor
# 示例:三角剖分五边形
n_sides = 5
polygon_points = polygon(n_sides)
triangulation = delaunay_lawson(polygon_points)
# 绘制结果
voronoi_plot_2d(triangulation)
plt.show()
阅读全文
相关推荐
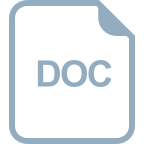
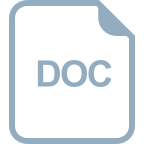
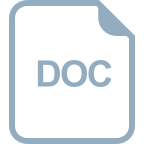

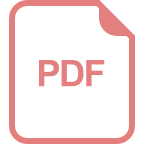
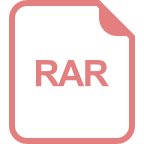
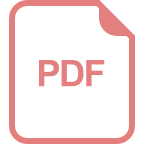
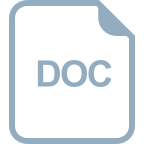
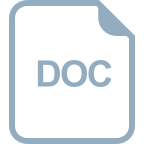
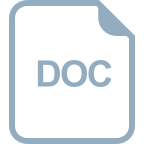
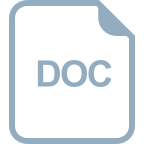
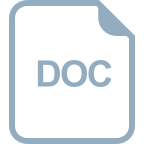



