写一个基于链表的一元多项式运算程序要有菜单,C语言实现
时间: 2024-10-17 14:04:11 浏览: 64
在C语言中,你可以创建一个基于链表的数据结构来存储一元多项式的系数和对应的变量指数,然后编写一个包含菜单驱动操作的程序来进行加法、减法、乘法等运算。下面是一个简单的示例,展示如何实现这个程序的基本框架:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Term {
double coefficient;
int exponent;
struct Term* next;
} Term;
// 创建新节点函数
Term* createTerm(double coef, int exp) {
Term* new_term = (Term*)malloc(sizeof(Term));
if (new_term == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
new_term->coefficient = coef;
new_term->exponent = exp;
new_term->next = NULL;
return new_term;
}
// 添加项到链表函数
void addTerm(Term** head, double coef, int exp) {
Term* new_term = createTerm(coef, exp);
if (new_term != NULL) {
if (*head == NULL) {
*head = new_term;
} else {
Term* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = new_term;
}
}
}
// 遍历并打印链表函数
void printPolynomial(Term* head) {
Term* temp = head;
while (temp != NULL) {
printf("%.2f*x^%d ", temp->coefficient, temp->exponent);
temp = temp->next;
}
printf("\n");
}
int main() {
Term* polynomial = NULL;
int choice;
char input[50];
do {
printf("Polynomial Calculator\n");
printf("1. Add term\n2. Subtract term\n3. Multiply polynomials\n4. Print polynomial\n5. Exit\n");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Enter coefficient and exponent (separated by space): ");
scanf("%lf %d", &input[0], &input[2]);
addTerm(&polynomial, atof(input), atoi(input + 2)); // Convert string to double and int
break;
case 2:
// Implement subtraction here (similar to addition)
break;
case 3:
// Implement multiplication of two polynomials here
break;
case 4:
printPolynomial(polynomial);
break;
case 5:
printf("Exiting...\n");
break;
default:
printf("Invalid choice. Please try again.\n");
break;
}
} while (choice != 5);
return 0;
}
```
在这个例子中,我们首先定义了一个`Term`结构体表示单个项,并实现了创建节点、添加项以及打印链表的操作。主循环中根据用户选择的不同执行相应的操作。
请注意,此代码仅展示了基础功能,实际的乘法操作需要额外处理,并且上述代码没有包括错误处理,例如输入验证。在实际应用中,你需要对代码进行完善。以下是几个相关问题:
1. 如何实现两个链表的乘法运算?
2. 如果我想删除链表中的某个特定项,应该怎么做?
3. 这段代码能处理负数系数吗?如果不能,怎么修改?
阅读全文
相关推荐



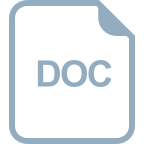














