一元多项式运算器减法C语言
时间: 2024-09-10 22:01:16 浏览: 98
一元多项式运算器实现减法操作通常涉及到对多项式链表的遍历和节点的合并。首先,我们需要定义多项式的节点结构,然后实现两个多项式的相减逻辑。下面是一个简化的例子来说明这一过程:
首先,定义一元多项式的节点结构:
```c
typedef struct PolyNode {
int coef; // 系数
int exp; // 指数
struct PolyNode *next; // 指向下一个节点的指针
} PolyNode, *Polynomial;
```
接下来,实现两个多项式的减法操作:
```c
Polynomial PolySub(Polynomial poly1, Polynomial poly2) {
Polynomial result = NULL; // 结果多项式
Polynomial temp1 = poly1, temp2 = poly2;
while (temp1 != NULL && temp2 != NULL) {
if (temp1->exp > temp2->exp) {
// 如果temp1的指数大于temp2的指数,则将temp1节点加入结果多项式
PolyInsert(&result, temp1->coef, temp1->exp);
temp1 = temp1->next;
} else if (temp1->exp < temp2->exp) {
// 如果temp2的指数大于temp1的指数,则将temp2节点的系数取反后加入结果多项式
PolyInsert(&result, -temp2->coef, temp2->exp);
temp2 = temp2->next;
} else {
// 如果两个节点的指数相同,则计算系数差,并根据差值是否为0决定是否加入结果多项式
int diff = temp1->coef - temp2->coef;
if (diff != 0) {
PolyInsert(&result, diff, temp1->exp);
}
temp1 = temp1->next;
temp2 = temp2->next;
}
}
// 将剩余的节点加入结果多项式
while (temp1 != NULL) {
PolyInsert(&result, temp1->coef, temp1->exp);
temp1 = temp1->next;
}
while (temp2 != NULL) {
PolyInsert(&result, -temp2->coef, temp2->exp);
temp2 = temp2->next;
}
return result;
}
```
这里的`PolyInsert`函数是一个辅助函数,用于将新节点正确地插入到结果多项式中,它需要确保结果多项式的节点是按照指数降序排列的。
需要注意的是,上述代码是一个简单的示例,并没有处理多项式的内存释放等细节。在实际应用中,还需要考虑内存管理,以及可能出现的特殊情况处理。
阅读全文
相关推荐
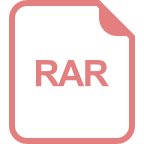
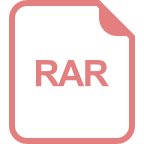
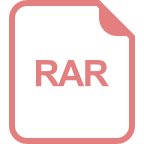















