一个旋转矩形绕矩形外未知的某一点旋转,怎么确定哪一边靠近旋转点,哪一边远离旋转点,用c++举例
时间: 2024-10-29 11:13:36 浏览: 22
当你有一个矩形围绕其外部的一个未知中心点进行旋转时,为了确定哪一侧更接近旋转点,哪一侧更远离,你可以通过计算每个顶点相对于旋转中心的距离,然后比较这些距离。如果一个顶点的绝对距离最小,那么对应的边就最接近旋转点;相反,如果另一个顶点的绝对距离最大,则相应的边远离旋转点。
以下是一个简单的 C++ 示例来说明这个过程:
```cpp
#include <cmath>
#include <iostream>
struct Point {
double x;
double y;
// 计算点到旋转中心的距离
double distanceToOrigin(double centerX, double centerY) const {
return std::sqrt(std::pow(x - centerX, 2) + std::pow(y - centerY, 2));
}
};
// 矩形类,包含四个顶点
class Rectangle {
public:
Point vertices[4];
// 假设旋转中心已知为originX, originY
void rotateAroundPoint(double originX, double originY, double rotationAngleInDegrees) {
// 先将角度转换为弧度
double rotationAngle = rotationAngleInDegrees * M_PI / 180.0;
// 对于每个顶点,计算旋转后的坐标并更新距离
for (int i = 0; i < 4; ++i) {
Point rotatedVert = {vertices[i].x * cos(rotationAngle) - vertices[i].y * sin(rotationAngle),
vertices[i].x * sin(rotationAngle) + vertices[i].y * cos(rotationAngle)};
vertices[i] = rotatedVert;
// 更新距离信息
vertices[i].distanceToOrigin = vertices[i].distanceToOrigin(originX, originY);
}
// 找出距离最小和最大的顶点,它们分别对应最近和最远的一边
int closestIndex = std::min_element(vertices, vertices + 4, [](const Point& a, const Point& b) -> bool {
return a.distanceToOrigin < b.distanceToOrigin;
});
int farthestIndex = std::max_element(vertices, vertices + 4, [](const Point& a, const Point& b) -> bool {
return a.distanceToOrigin > b.distanceToOrigin;
});
std::cout << "Closest side: (" << vertices[*closestIndex].x << ", " << vertices[*closestIndex].y << ")" << std::endl;
std::cout << "Farthest side: (" << vertices[*farthestIndex].x << ", " << vertices[*farthestIndex].y << ")" << std::endl;
}
};
int main() {
// 创建矩形并旋转
Rectangle rect;
// ... set rectangle vertices
rect.rotateAroundPoint(0, 0, 90); // 旋转90度示例
return 0;
}
```
这个程序首先计算每个顶点经过旋转后的坐标,然后根据距离来判断哪一边靠近旋转点,哪一边远离。注意这只是一个基本的示例,实际应用中可能需要处理更多的边缘情况,如边界条件、精度问题等。
阅读全文
相关推荐



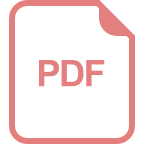














