用c++实现求n的阶乘,要求使用递归与非递归两种方法。 。编程实现求斐波那契数列的前20个数,要求每行输出5个数据,即每输出5个数即换行
时间: 2024-10-22 09:06:07 浏览: 34
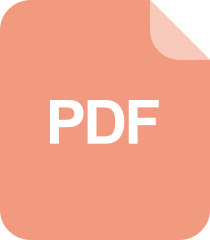
C++基于递归算法解决汉诺塔问题与树的遍历功能示例

首先,让我们分别用C++实现计算n的阶乘的递归和非递归方法。
**递归方法** (计算阶乘):
```cpp
#include <iostream>
using namespace std;
// 递归函数计算阶乘
unsigned long long factorial_recursive(int n) {
if (n == 0 || n == 1)
return 1;
else
return n * factorial_recursive(n - 1);
}
int main() {
int n;
cout << "请输入一个整数: ";
cin >> n;
unsigned long long result = factorial_recursive(n);
cout << "阶乘结果: " << result << endl;
return 0;
}
```
**非递归方法** (计算阶乘):
```cpp
#include <iostream>
using namespace std;
// 非递归函数计算阶乘
unsigned long long factorial_iterative(int n) {
unsigned long long fact = 1;
for (int i = 1; i <= n; ++i) {
fact *= i;
}
return fact;
}
int main() {
int n;
cout << "请输入一个整数: ";
cin >> n;
unsigned long long result = factorial_iterative(n);
cout << "阶乘结果: " << result << endl;
return 0;
}
```
接下来,我们来编写一个C++程序,计算并打印斐波那契数列的前20个数,每行输出5个数据:
```cpp
#include <iostream>
// 斐波那契数列生成器
void fibonacci(int n) {
int a = 0, b = 1, c;
for (int i = 0; i < n; ++i) {
if (i % 5 == 0 && i > 0) { // 每隔5个数换行
cout << "\n";
}
cout << c << " ";
c = a + b;
a = b;
b = c;
}
cout << endl;
}
int main() {
fibonacci(20);
return 0;
}
```
阅读全文
相关推荐
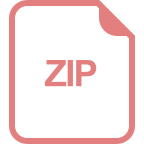
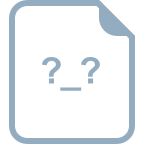
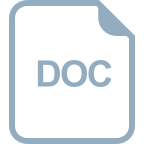
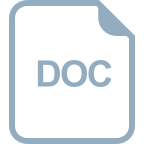
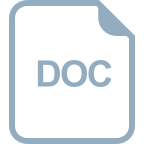
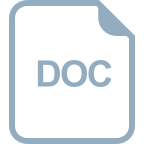
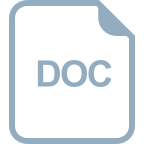
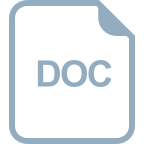
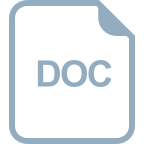
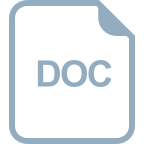
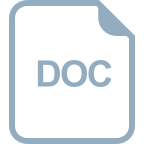
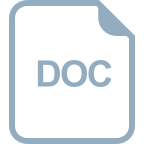
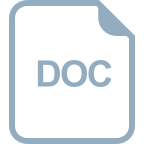
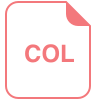



