c语言编写一个加密程序实现广义Caesar密码
时间: 2024-09-22 19:04:17 浏览: 39
在C语言中,广义Caesar密码是一种简单的替换式加密技术,通过将明文字母按照一定的偏移量向右或向左移动来加密。这里是一个基本的广义Caesar密码加密和解密程序示例:
```c
#include <stdio.h>
#include <string.h>
// 加密函数
char caesar_encrypt(char char_to_encrypt, int shift) {
if (char_to_encrypt >= 'A' && char_to_encrypt <= 'Z') { // 大写字母
return ((char_to_encrypt - 'A' + shift) % 26) + 'A';
} else if (char_to_encrypt >= 'a' && char_to_encrypt <= 'z') { // 小写字母
return ((char_to_encrypt - 'a' + shift) % 26) + 'a';
}
return char_to_encrypt; // 对于非字母字符不做处理
}
// 解密函数
char caesar_decrypt(char encrypted_char, int shift) {
return caesar_encrypt(encrypted_char, -shift);
}
int main() {
char plaintext[100], ciphertext[100];
int shift;
printf("请输入明文:\n");
fgets(plaintext, sizeof(plaintext), stdin); // 跳过换行符
plaintext[strlen(plaintext)-1] = '\0'; // 去掉fgets读取的换行符
printf("请输入偏移量(整数): ");
scanf("%d", &shift);
for (int i = 0; plaintext[i]; ++i) {
ciphertext[i] = caesar_encrypt(plaintext[i], shift);
}
ciphertext[strlen(ciphertext)] = '\0';
printf("加密后的密文: %s\n", ciphertext);
// 如果需要解密,只需改变加密函数调用中的偏移量方向
// printf("请输入要解密的密文:\n");
// fgets(ciphertext, sizeof(ciphertext), stdin);
// ciphertext[strlen(ciphertext)-1] = '\0';
// printf("解密后的原文: %s\n", caesar_decrypt(ciphertext, -shift)); // 使用caesar_decrypt函数
return 0;
}
```
这个程序会提示用户输入明文、偏移量,并对明文字母进行加密。如果需要,还可以添加解密功能。
阅读全文
相关推荐
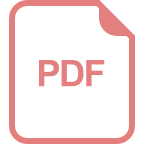
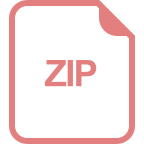


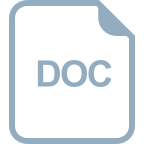

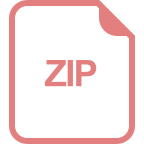
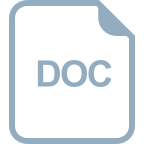
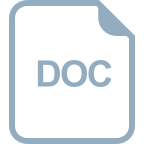
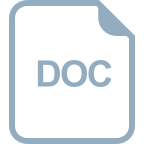
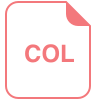







