如何用C语言编写一个简单的密码转换
时间: 2024-10-27 16:13:36 浏览: 29
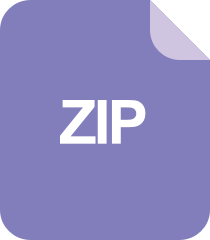
C语言简单凯撒密码.zip
在C语言中,我们可以编写一个简单的密码转换程序,比如将明文字符通过某种规则转化为密文。这里我将以凯撒密码(Caesar Cipher)为例,它是一种替换加密技术,每个字母向后移动固定位数。
下面是一个简单的凯撒密码加密函数的示例:
```c
#include <stdio.h>
#include <ctype.h>
char shift(char c, int key) {
// 检查字符是否为大写字母或小写字母
if (isalpha(c)) {
// ASCII码中'A'到'Z'(大写)对应97到122,'a'到'z'(小写)对应65到90
if (isupper(c))
return ((c - 'A' + key) % 26) + 'A';
else
return ((c - 'a' + key) % 26) + 'a';
}
// 非字母字符保持不变
return c;
}
void caesar_encrypt(char *plaintext, int key, char *ciphertext) {
int i;
for (i = 0; plaintext[i] != '\0'; i++) {
ciphertext[i] = shift(plaintext[i], key);
}
ciphertext[i] = '\0'; // 添加终止符
}
int main() {
char plaintext[100], ciphertext[100];
printf("请输入明文:\n");
fgets(plaintext, sizeof(plaintext), stdin); // 读入一行
plaintext[strlen(plaintext) - 1] = '\0'; // 去除换行符
int key;
printf("请输入偏移量(key): ");
scanf("%d", &key);
caesar_encrypt(plaintext, key, ciphertext);
printf("加密后的密文是: %s\n", ciphertext);
return 0;
}
```
在这个程序中,`caesar_encrypt` 函数接收明文、偏移量(key),并将明文中的每个字符按照凯撒密码规则进行转换。然后在`main`函数中获取用户输入并展示加密结果。
阅读全文
相关推荐
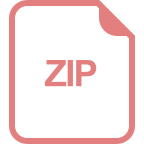
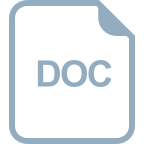



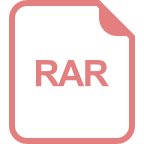






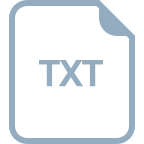
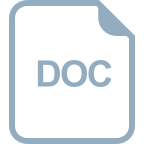
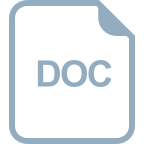


