如何设计一个掷骰子游戏(两个人同时掷俩骰子并比大小)的类图,并给出示例
时间: 2024-11-09 14:23:17 浏览: 18
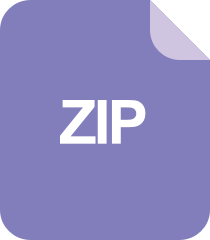
Java编写掷骰子游戏共4页.pdf.zip
设计一个掷骰子游戏的类图,我们可以构建以下几类:
1. **Dice**类: 表示单个骰子,包含骰子面数(默认6面)和当前显示的点数。它有一个roll()方法用于随机生成点数。
```python
class Dice:
def __init__(self):
self.faces = 6
self.current_roll = None
def roll(self):
self.current_roll = random.randint(1, self.faces)
```
2. **Player**类: 包含姓名和两个Dice实例,用于表示玩家拥有的骰子。
```python
class Player:
def __init__(self, name):
self.name = name
self.dice_1 = Dice()
self.dice_2 = Dice()
```
3. **Game**类: 管理比赛流程,包括两个玩家,比较点数和结果。它可能包含一个play_round()方法来进行一轮掷骰子和比较。
```python
class Game:
def __init__(self, player1, player2):
self.player1 = player1
self.player2 = player2
def play_round(self):
self.player1.dice_1.roll()
self.player1.dice_2.roll()
self.player2.dice_1.roll()
self.player2.dice_2.roll()
if self.compare_scores(self.player1) > self.compare_scores(self.player2):
print(f"{self.player1.name} wins this round!")
else:
print(f"{self.player2.name} wins this round!")
def compare_scores(self, player):
return sum(player.dice_1.current_roll, player.dice_2.current_roll)
```
4. **Scoreboard**类: 可以用于记录每轮的结果和整个比赛的总分。
```python
class Scoreboard:
def __init__(self, game):
self.game = game
self.round_scores = []
def record_score(self, winner):
self.round_scores.append((winner, self.game.play_round()))
```
阅读全文
相关推荐
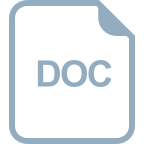
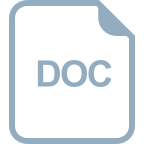
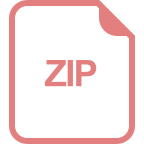
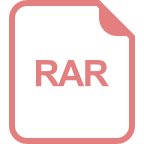
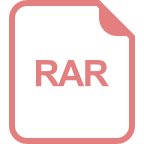
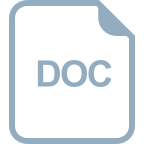
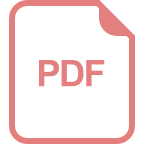
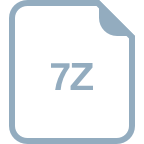
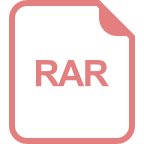
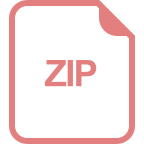
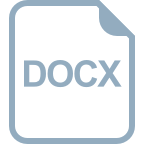
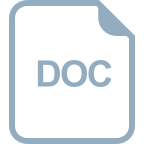




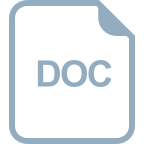