随机森林 java代码
时间: 2024-11-21 11:28:53 浏览: 12
随机森林是一种集成学习算法,通过构建多个决策树并取其平均结果来提高预测性能。在Java中,可以使用Apache Mahout、Weka或者自家实现的库来编写随机森林模型。下面是一个简单的使用Weka库创建随机森林的例子:
```java
import weka.classifiers.trees.RandomForest;
import weka.core.Instances;
// 加载数据集
Instances data = ... //从CSV文件或数据库读取
data.setClassIndex(data.numAttributes() - 1); //假设最后一列是目标变量
// 创建RandomForest分类器
RandomForest randomForest = new RandomForest();
randomForest.setNumTrees(100); //设置树的数量
randomForest.buildClassifier(data);
// 使用模型进行预测
double[] predictions = randomForest.distributionForInstance(new DenseInstance(data.numAttributes(), data.instance(i).toDoubleArray()));
```
在这个例子中,首先加载数据,然后实例化一个`RandomForest`对象,并设置所需的参数(如树的数量)。接着,用训练数据对模型进行训练,最后用模型对新的实例进行预测。
相关问题
java 随机森林算法代码
下面是一个简单的Java随机森林算法的实现代码示例:
```
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Random;
public class RandomForest {
private List<DecisionTree> forest;
private int numTrees;
private int maxDepth;
private int minLeafSize;
private int numFeatures;
public RandomForest(int numTrees, int maxDepth, int minLeafSize, int numFeatures) {
this.numTrees = numTrees;
this.maxDepth = maxDepth;
this.minLeafSize = minLeafSize;
this.numFeatures = numFeatures;
this.forest = new ArrayList<DecisionTree>();
}
public void train(List<DataPoint> data) {
int numDataPoints = data.size();
for (int i = 0; i < numTrees; i++) {
// Randomly select a subset of features
List<Integer> featureIndices = new ArrayList<Integer>();
for (int j = 0; j < numFeatures; j++) {
featureIndices.add(j);
}
Collections.shuffle(featureIndices);
featureIndices = featureIndices.subList(0, numFeatures);
// Randomly select a subset of data points
List<DataPoint> subset = new ArrayList<DataPoint>();
for (int j = 0; j < numDataPoints; j++) {
int index = new Random().nextInt(numDataPoints);
subset.add(data.get(index));
}
// Build a decision tree using the selected features and data points
DecisionTree tree = new DecisionTree(maxDepth, minLeafSize, featureIndices);
tree.train(subset);
forest.add(tree);
}
}
public int predict(DataPoint data) {
int numTrees = forest.size();
int[] predictions = new int[numTrees];
for (int i = 0; i < numTrees; i++) {
predictions[i] = forest.get(i).predict(data);
}
// Return the mode of the predictions
int mode = predictions[0];
int maxCount = 0;
for (int i = 0; i < numTrees; i++) {
int count = 0;
for (int j = 0; j < numTrees; j++) {
if (predictions[j] == predictions[i]) {
count++;
}
}
if (count > maxCount) {
mode = predictions[i];
maxCount = count;
}
}
return mode;
}
}
```
请注意,上述代码中的 `DataPoint` 和 `DecisionTree` 类并未给出,因此你需要根据自己的数据结构实现它们。此外,这个实现仅作为参考,你可以根据自己的需求进行修改和改进。
随机森林 java实现
在Java中实现随机森林,可以使用Weka这个机器学习框架。Weka提供了RandomForest类,可以用来构建随机森林模型。以下是一个简单的示例代码:
```java
import weka.classifiers.trees.RandomForest;
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
public class RandomForestExample {
public static void main(String[] args) throws Exception {
// 加载数据集
DataSource source = new DataSource("path/to/your/dataset.arff");
Instances data = source.getDataSet();
if (data.classIndex() == -1) {
data.setClassIndex(data.numAttributes() - 1);
}
// 构建随机森林模型
RandomForest rf = new RandomForest();
rf.buildClassifier(data);
// 输出模型
System.out.println(rf);
}
}
```
在这个例子中,我们首先加载了一个数据集,然后用RandomForest类构建了一个随机森林模型。最后,我们将模型输出到控制台。你可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐
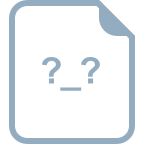
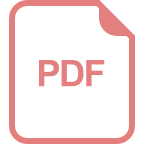
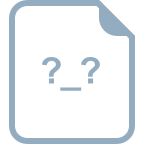













