C语言将字符串大写转小写小写变大写
时间: 2024-09-12 18:10:18 浏览: 67
在C语言中,没有内置函数可以直接完成字符串的大写转小写或小写转大写的转换。然而,你可以通过遍历字符串并逐个字符处理来实现这个功能。这里提供一种常见的手动转换的方法:
**大写转小写**:
```c
#include <ctype.h>
#include <string.h>
void to_lower(char *str) {
int len = strlen(str);
for (int i = 0; i < len; i++) {
if (isupper(str[i])) { // 判断字符是否为大写字母
str[i] = tolower(str[i]); // 转换为小写
}
}
}
```
**小写转大写**:
```c
#include <ctype.h>
#include <string.h>
void to_upper(char *str) {
int len = strlen(str);
for (int i = 0; i < len; i++) {
if (islower(str[i])) { // 判断字符是否为小写字母
str[i] = toupper(str[i]); // 转换为大写
}
}
}
```
注意,`isupper()` 和 `tolower()`、`islower()` 和 `toupper()` 分别用于判断字符是否为大写或小写,并进行相应的转换。
相关问题
c语言将字符串大写转小写
在C语言中,将字符串从大写转换成小写并不直接内置一个函数,因为这是大小写的字符映射操作,需要遍历每个字符并逐个修改。你可以通过以下步骤手动实现:
1. 定义一个ASCII值范围,A-Z对应于65到90的小写字母a-z,它们之间有一个固定的偏移量。
2. 遍历输入字符串,对于每个大写字母(ASCII码大于64),将其减去这个偏移量,得到相应的小写字母。
3. 使用C语言的标准库函数如`tolower()`(如果可用的话)来进行更便捷的转换,但这通常只适用于预定义的字符集,而非整个字符串。
下面是一个简单的示例,使用`isupper()`检查字符是否为大写,并用`tolower()`进行转换:
```c
#include <ctype.h>
#include <stdio.h>
char *to_lower(char *str) {
while (*str) {
if (isupper(*str)) {
*str = tolower(*str);
}
str++;
}
return str;
}
int main() {
char str[] = "HELLO WORLD";
str = to_lower(str); // 转换后的结果现在存储在str数组中
printf("%s\n", str); // 输出:hello world
return 0;
}
```
请注意,这种方法仅对ASCII字符有效,对于Unicode字符串,处理起来会复杂一些。
c语言字符串大写转小写
C语言中可以使用库函数`tolower()`将大写字母转换为小写字母。可以使用循环遍历字符串中的每个字符,然后将大写字母转换为小写字母。以下是一个示例代码:
```c
#include <stdio.h>
#include <ctype.h>
void toLower(char *str) {
int i = 0;
while (str[i]) {
str[i] = tolower(str[i]);
i++;
}
}
int main() {
char str[] = "HELLO WORLD";
printf("Before: %s\n", str);
toLower(str);
printf("After: %s\n", str);
return 0;
}
```
输出结果为:
```
Before: HELLO WORLD
After: hello world
```
阅读全文
相关推荐
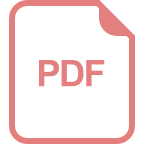
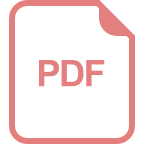
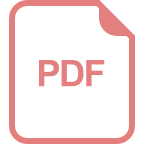












