opencv棋盘格鸟瞰图
时间: 2023-07-31 18:07:08 浏览: 63
对于使用OpenCV处理棋盘格鸟瞰图,你可以按照以下步骤进行操作:
1. 首先,使用OpenCV的摄像头校准功能来获取相机的内部参数和畸变系数。这可以通过拍摄一系列棋盘格图像并使用`cv2.calibrateCamera()`函数来完成。
2. 然后,在获取到相机的内部参数后,你可以使用`cv2.undistort()`函数来校正图像的畸变。
3. 接下来,通过对校正后的图像进行透视变换,将其转换为鸟瞰图。透视变换需要定义一个转换矩阵,可以通过OpenCV的`cv2.getPerspectiveTransform()`函数来获得。
4. 使用获得的透视变换矩阵,可以使用`cv2.warpPerspective()`函数将图像转换为鸟瞰图。
下面是一个示例代码,展示了如何使用OpenCV处理棋盘格鸟瞰图:
```python
import cv2
import numpy as np
# 标定棋盘格参数
rows = 6
cols = 9
square_size = 1
# 棋盘格角点坐标
objp = np.zeros((rows*cols, 3), np.float32)
objp[:, :2] = np.mgrid[0:cols, 0:rows].T.reshape(-1, 2) * square_size
# 存储棋盘格角点的世界坐标和图像坐标
objpoints = [] # 世界坐标
imgpoints = [] # 图像坐标
# 根据摄像头拍摄的棋盘格图像进行标定
def calibrate_camera():
# 读取棋盘格图像
images = glob.glob('chessboard/*.jpg')
for fname in images:
img = cv2.imread(fname)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 寻找棋盘格角点
ret, corners = cv2.findChessboardCorners(gray, (cols, rows), None)
if ret == True:
objpoints.append(objp)
imgpoints.append(corners)
# 校准相机
ret, mtx, dist, rvecs, tvecs = cv2.calibrateCamera(objpoints, imgpoints, gray.shape[::-1], None, None)
return mtx, dist
# 校正图像的畸变
def undistort_image(image, mtx, dist):
undistorted_img = cv2.undistort(image, mtx, dist, None, mtx)
return undistorted_img
# 获取透视变换矩阵
def get_perspective_transform(image):
# 定义棋盘格四个角点的像素坐标
src_points = np.float32([(x, y) for y in range(0, rows) for x in range(0, cols)])
# 定义鸟瞰图四个角点的像素坐标
dst_points = np.float32([[0, 0], [cols-1, 0], [cols-1, rows-1], [0, rows-1]])
# 计算透视变换矩阵
M = cv2.getPerspectiveTransform(src_points, dst_points)
return M
# 将图像转换为鸟瞰图
def transform_to_birdseye(image, M):
birdseye_img = cv2.warpPerspective(image, M, (cols, rows))
return birdseye_img
# 主程序
if __name__ == '__main__':
# 读取原始图像
image = cv2.imread('chessboard_image.jpg')
# 相机校准
mtx, dist = calibrate_camera()
# 校正图像的畸变
undistorted_img = undistort_image(image, mtx, dist)
# 获取透视变换矩阵
M = get_perspective_transform(undistorted_img)
# 将图像转换为鸟瞰图
birdseye_img = transform_to_birdseye(undistorted_img, M)
# 显示结果
cv2.imshow('Original Image', image)
cv2.imshow('Undistorted Image', undistorted_img)
cv2.imshow('Birdseye Image', birdseye_img)
cv2.waitKey(0)
```
请确保你已经准备好了标定棋盘格图像,并将其存储在`chessboard/`文件夹中,原始图像命名为`chessboard_image.jpg`。你可以根据自己的需求修改代码中的参数和路径。该代码将显示原始图像、校正后的图像和鸟瞰图。
相关推荐
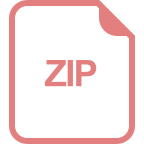














