发送ble广播 聊天 源码
时间: 2023-09-01 15:05:23 浏览: 171
发送BLE广播聊天的源码示例如下:
```java
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothGattCharacteristic;
import android.bluetooth.BluetoothGattService;
import android.content.Context;
import android.os.Bundle;
import android.os.Handler;
import android.os.Looper;
import android.os.Message;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
public class ChatService {
private Context mContext;
private BluetoothAdapter mBluetoothAdapter;
private Handler mHandler = new Handler(Looper.getMainLooper()) {
@Override
public void handleMessage(Message msg) {
switch (msg.what) {
case MESSAGE_READ:
byte[] buffer = (byte[]) msg.obj;
String message = new String(buffer, 0, msg.arg1);
// 处理接收到的消息
break;
}
}
};
public ChatService(Context context) {
mContext = context;
mBluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
}
public void startAdvertising() {
BluetoothGattService gattService = new BluetoothGattService(
UUID.fromString("0000xxxx-0000-1000-8000-00805F9B34FB"),
BluetoothGattService.SERVICE_TYPE_PRIMARY);
BluetoothGattCharacteristic characteristic = new BluetoothGattCharacteristic(
UUID.fromString("0000yyyy-0000-1000-8000-00805F9B34FB"),
BluetoothGattCharacteristic.PROPERTY_READ | BluetoothGattCharacteristic.PROPERTY_WRITE,
BluetoothGattCharacteristic.PERMISSION_READ | BluetoothGattCharacteristic.PERMISSION_WRITE);
gattService.addCharacteristic(characteristic);
List<BluetoothGattService> gattServices = new ArrayList<>();
gattServices.add(gattService);
mBluetoothAdapter.getBluetoothLeAdvertiser().startAdvertising(
createAdvertiseSettings(true),
createAdvertiseData(gattServices),
mAdvertiseCallback);
}
private AdvertiseCallback mAdvertiseCallback = new AdvertiseCallback() {
@Override
public void onStartSuccess(AdvertiseSettings settingsInEffect) {
// 广播开始成功
}
@Override
public void onStartFailure(int errorCode) {
// 广播开始失败
}
};
private AdvertiseSettings createAdvertiseSettings(boolean connectable) {
AdvertiseSettings.Builder builder = new AdvertiseSettings.Builder();
builder.setAdvertiseMode(AdvertiseSettings.ADVERTISE_MODE_BALANCED);
builder.setConnectable(connectable);
builder.setTimeout(0);
builder.setTxPowerLevel(AdvertiseSettings.ADVERTISE_TX_POWER_MEDIUM);
return builder.build();
}
private AdvertiseData createAdvertiseData(List<BluetoothGattService> services) {
AdvertiseData.Builder builder = new AdvertiseData.Builder();
for (BluetoothGattService service : services) {
builder.addServiceUuid(new ParcelUuid(service.getUuid()));
}
return builder.build();
}
}
```
上述代码实现了手机作为BLE外设发送广播聊天功能。通过`startAdvertising()`方法开启广播,使用自定义的UUID创建Gatt服务和特征,并将其添加到广播数据中。当其他设备扫描并连接上该广播时,可以读取特定的Gatt特征,实现聊天功能。在`handleMessage()`方法中处理接收到的消息。
阅读全文
相关推荐
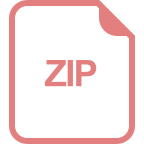
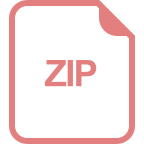















