unity 需要向下位机发送串口命令,需要等待下位机处理反馈,然后再执行新的动作,写个通用函数
时间: 2024-11-15 18:25:08 浏览: 12
在Unity中,如果你需要通过串口与下位机通信并处理其响应,通常会使用`System.IO.Ports.SerialPort`类,假设我们想创建一个通用的函数来实现这个功能。以下是一个简单的示例:
```csharp
using System.IO.Ports;
using UnityEngine;
public class SerialCommunication : MonoBehaviour
{
private const string DEFAULT_PORT_NAME = "COM1"; // 这里替换为你实际使用的端口号
private const int BUFFER_SIZE = 1024;
private SerialPort serialPort;
void Start()
{
serialPort = new SerialPort(DEFAULT_PORT_NAME, 9600); // 设置波特率、数据位等配置
serialPort.Open();
}
public IEnumerator SendAndReceiveCommand(string command, Action<string> onFeedbackReceived)
{
try
{
// 发送命令
serialPort.Write(command + "\r\n");
// 接收反馈,直到收到换行符
byte[] buffer = new byte[BUFFER_SIZE];
int receivedBytes = 0;
while (receivedBytes < 2) // 确保接收到了完整的反馈(包括回车)
{
receivedBytes += serialPort.Read(buffer, receivedBytes, BUFFER_SIZE - receivedBytes);
}
// 提取反馈字符串
string feedback = System.Text.Encoding.ASCII.GetString(buffer, 0, receivedBytes - 1).Trim();
// 如果有回调函数,则执行
if (onFeedbackReceived != null)
{
onFeedbackReceived(feedback);
}
yield return null; // 等待一段时间或者直到接收到特定的结束信号,然后继续执行下一个动作
}
catch (Exception ex)
{
Debug.LogError("Error sending/receiving command: " + ex.Message);
}
finally
{
// 关闭连接以释放资源
if (serialPort.IsOpen)
{
serialPort.Close();
}
}
}
// 示例调用:
public void ExecuteNextActionAfterFeedback()
{
StartCoroutine(SendAndReceiveCommand("your_command", OnFeedbackProcessed));
}
private void OnFeedbackProcessed(string feedback)
{
// 根据反馈做后续操作...
Debug.Log($"Received feedback: {feedback}");
// ...在这里添加你需要的下一步动作
}
}
```
在这个例子中,`SendAndReceiveCommand`函数接受一个命令字符串和一个回调方法`onFeedbackReceived`,它会在收到下位机反馈后执行该回调。注意这是一个基本框架,你需要根据实际情况调整等待时间,错误处理以及反馈解析部分。
阅读全文
相关推荐
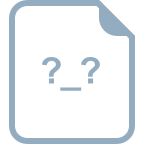
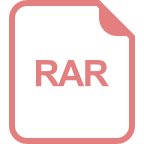
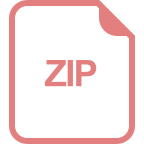






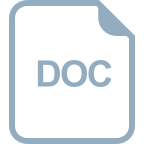
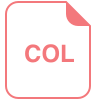
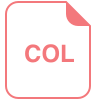
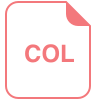
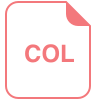
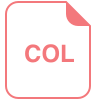
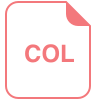
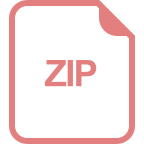
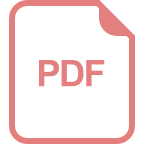
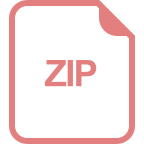