解释一下代码 for j, d in enumerate(data_list): lat, lon = d['lat'], d['lon'] value = data_type[j] color = cmap(value / max(data_type)) ax.scatter(lon, lat, s=100, color=color, alpha=1.0)
时间: 2024-04-17 09:27:26 浏览: 17
这段代码是一个循环,用于绘制散点图。它遍历了一个名为data_list的数据列表,并使用enumerate函数获取索引和对应的元素。
在每次循环中,代码从当前元素d中提取经度(lon)和纬度(lat)的值。接下来,它从data_type列表中获取相应索引位置的值作为散点的数值。
然后,代码根据数值与data_type中的最大值比例,使用cmap函数来确定散点的颜色。cmap是一个颜色映射函数,它将数值映射到一组颜色中。
最后,代码使用matplotlib库(ax.scatter函数)绘制一个散点,位置由经度(lon)和纬度(lat)决定。散点的大小(s)为100,颜色(color)根据前面计算得到的color变量确定,透明度(alpha)为1.0。
相关问题
# 准备画轮廓图的数据点 X, Y = np.meshgrid(xgrid[::5], ygrid[::5][::-1]) land_reference = data.coverages[6][::5, ::5] land_mask = (land_reference > -9999).ravel() xy = np.vstack([Y.ravel(), X.ravel()]).T xy = np.radians(xy[land_mask]) # 创建两幅并排的图 fig, ax = plt.subplots(1, 2) fig.subplots_adjust(left=0.05, right=0.95, wspace=0.05) species_names = ['Bradypus Variegatus', 'Microryzomys Minutus'] cmaps = ['Purples', 'Reds'] for i, axi in enumerate(ax): axi.set_title(species_names[i]) # 用Basemap画出海岸线 m = Basemap(projection='cyl', llcrnrlat=Y.min(), urcrnrlat=Y.max(), llcrnrlon=X.min(), urcrnrlon=X.max(), resolution='c', ax=axi) m.drawmapboundary(fill_color='#DDEEFF') m.drawcoastlines() m.drawcountries() # 构建一个球形的分布核密度估计 kde = KernelDensity(bandwidth=0.03, metric='haversine') kde.fit(np.radians(latlon[species == i])) # 只计算大陆的值:-9999表示是海洋 Z = np.full(land_mask.shape[0], -9999.0) Z[land_mask] = np.exp(kde.score_samples(xy)) Z = Z.reshape(X.shape) # 画出密度的轮廓 levels = np.linspace(0, Z.max(), 25) axi.contourf(X, Y, Z, levels=levels, cmap=cmaps[i])
这段代码使用了 Matplotlib 库和 Basemap 库绘制了两幅并排的轮廓图。首先,使用 `np.meshgrid()` 函数生成了两个二维数组 `X` 和 `Y`,用于在 x 轴和 y 轴上绘制轮廓图的网格。然后,使用 `data.coverages[6][::5, ::5]` 获取了数据的第 6 个覆盖范围,并使用 `land_mask = (land_reference > -9999).ravel()` 创建了一个一维布尔数组,用于筛选出陆地上的数据点。接着,使用 `np.vstack()` 函数和 `np.radians()` 函数将筛选出的数据点转换为弧度制,并将其存储在 `xy` 变量中。接下来,使用 `Basemap()` 函数创建了一个地图投影,并使用 `m.drawmapboundary()`、`m.drawcoastlines()` 和 `m.drawcountries()` 函数绘制了地图边界、海岸线和国家边界。然后,使用 `KernelDensity()` 函数创建了一个球形分布的核密度估计,使用 `kde.fit()` 函数拟合了第一个物种的数据,并将其存储在 `Z` 变量中。接着,使用 `np.full()` 函数创建一个全为 -9999 的一维数组 `Z`,并将筛选出的陆地上的数据点的密度估计结果存储在 `Z` 中。然后,使用 `Z.reshape()` 函数将一维数组 `Z` 转换为二维数组,以匹配 `X` 和 `Y` 的形状。最后,使用 `axi.contourf()` 函数绘制了密度的轮廓,并使用 `cmaps[i]` 参数指定了颜色映射。
提取nc文件里(30N,100E)附近所有时间的数据,并利用write函数将数据输出到out_2mt.txt文件,要求第一列为时间,第二列为温度值。
由于不知道该nc文件中的具体变量和时间信息,以下是一个示例代码,仅供参考:
```python
import netCDF4 as nc
# 打开nc文件
data = nc.Dataset('filename.nc')
# 获取时间变量
time_var = data.variables['time']
times = nc.num2date(time_var[:], time_var.units)
# 获取经纬度变量
lat_var = data.variables['lat']
lon_var = data.variables['lon']
# 获取温度变量
temp_var = data.variables['temperature']
# 获取30N,100E附近的格点索引
lat_idx = (lat_var[:] >= 29.5) & (lat_var[:] <= 30.5)
lon_idx = (lon_var[:] >= 99.5) & (lon_var[:] <= 100.5)
idx = lat_idx & lon_idx
# 提取数据
times_2mt = []
temps_2mt = []
for i, time in enumerate(times):
temp = temp_var[i, idx]
if not temp.mask: # 如果该格点数据未缺失
times_2mt.append(time.strftime('%Y-%m-%d %H:%M:%S'))
temps_2mt.append(temp.data[0])
# 输出数据到文件
with open('out_2mt.txt', 'w') as f:
for time, temp in zip(times_2mt, temps_2mt):
f.write(f'{time}\t{temp:.2f}\n')
```
解释一下代码:
1. 打开nc文件并获取时间、经纬度、温度变量。
2. 获取30N,100E附近的格点索引(这里假设经纬度变量是一维的)。
3. 遍历所有时间,如果该时间的30N,100E的温度数据未缺失,则将时间和温度值加入对应的列表中。
4. 使用`with open()`语句打开文件,遍历时间和温度值列表,将其输出到文件中。
相关推荐
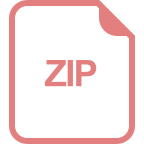
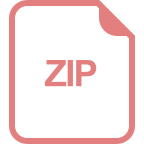
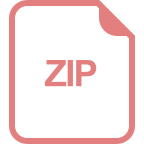












