构造一个容量为5的双向链表,结点结构为data,prior,next,其中各结点data域依次为:1,2,3,4,5。先p指向链表中第3各结点,编写chang(p)函数,将p所指向的结点与其前驱交换顺序、用c++写代码
时间: 2024-04-15 21:25:56 浏览: 86
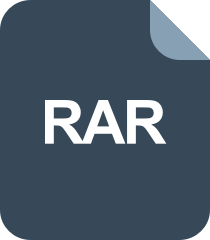
用C++实现双向链表

好的,以下是根据您的要求编写的C++代码:
```cpp
#include <iostream>
using namespace std;
struct Node {
int data;
Node* prior;
Node* next;
};
void change(Node* &p) {
if (p == nullptr || p->prior == nullptr || p->prior->prior == nullptr) {
// 不满足交换条件,直接返回
return;
}
Node* temp = p->prior->prior;
temp->next = p;
p->prior->next = p->next;
p->next = p->prior;
p->prior = temp;
temp->next->prior = temp;
}
int main() {
// 创建容量为5的双向链表
Node* head = nullptr;
Node* tail = nullptr;
for (int i = 1; i <= 5; i++) {
Node* newNode = new Node;
newNode->data = i;
newNode->prior = nullptr;
newNode->next = nullptr;
if (head == nullptr) {
head = newNode;
tail = newNode;
} else {
tail->next = newNode;
newNode->prior = tail;
tail = newNode;
}
}
// 找到p指向链表中第3个结点
Node* p = head->next->next;
// 输出交换前链表的顺序
cout << "交换前链表的顺序:";
for (Node* node = head; node != nullptr; node = node->next) {
cout << node->data << " ";
}
cout << endl;
// 调用change函数进行交换
change(p);
// 输出交换后链表的顺序
cout << "交换后链表的顺序:";
for (Node* node = head; node != nullptr; node = node->next) {
cout << node->data << " ";
}
cout << endl;
// 释放内存,防止内存泄漏
Node* current = head;
while (current != nullptr) {
Node* next = current->next;
delete current;
current = next;
}
return 0;
}
```
在这个代码中,我们首先定义了一个双向链表的结构体`Node`,其中包含了数据域`data`,前驱指针`prior`和后继指针`next`。
然后,我们实现了`change`函数,它接受一个指向链表中某个结点的指针`p`作为参数。在函数中,我们首先判断是否满足交换条件,即`p`指向的结点不是头结点或者前驱结点不是头结点。如果满足条件,我们进行结点交换操作,即通过修改各结点的指针来交换`p`和其前驱结点的顺序。
在`main`函数中,我们创建了容量为5的双向链表,并找到`p`指向链表中第3个结点。然后,我们调用`change`函数进行结点交换,并输出交换前后链表的顺序。
最后,我们释放链表中各结点的内存,防止内存泄漏。
阅读全文
相关推荐
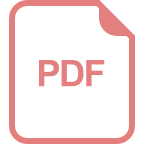














