c语言1. 定义单链表结构体,获取数据元素。 2. 创建链表以输入-1结束。 3. 在第i个节点处插入数据元素x。 4. 删除第j个节点。 5. 显示链表中的数据元素。 6. 逆序显示链表中的数据元素。一次性生成
时间: 2024-09-09 17:15:45 浏览: 99
在C语言中,我们可以使用结构体定义单链表,并完成上述操作。这里是一个简单的示例:
1. **定义单链表结构体**:
```c
typedef struct Node {
int data; // 数据域
struct Node* next; // 指针域,指向下一个节点
} Node;
```
2. **创建链表并输入-1结束**:
```c
Node* createList(int input[], int n) {
if (n <= 0) return NULL;
Node* head = malloc(sizeof(Node));
head->data = input[0];
head->next = NULL;
for (int i = 1; i < n; i++) {
Node* newNode = malloc(sizeof(Node));
newNode->data = input[i];
newNode->next = head->next;
head->next = newNode;
}
if (input[n - 1] != -1) { // 如果输入数组未以-1结尾,添加一个终止节点
Node* terminator = malloc(sizeof(Node));
terminator->data = -1;
terminator->next = NULL;
head->next = terminator;
}
return head;
}
```
3. **在第i个节点处插入数据元素x**:
```c
void insertAtPosition(Node** head, int index, int x) {
if (index <= 0) return;
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = x;
if (*head == NULL || index == 1) {
newNode->next = *head;
*head = newNode;
} else {
Node* current = *head;
for (int i = 1; i < index && current != NULL; i++)
current = current->next;
newNode->next = current->next;
current->next = newNode;
}
}
```
4. **删除第j个节点**:
```c
void deleteNodeAtPosition(Node** head, int position) {
if (*head == NULL || position <= 0) return;
if (position == 1) {
Node* temp = *head;
*head = temp->next;
free(temp);
} else {
Node* current = *head;
Node* previous = NULL;
for (int i = 1; i < position && current != NULL; i++) {
previous = current;
current = current->next;
}
if (current != NULL) {
previous->next = current->next;
free(current);
}
}
}
```
5. **显示链表中的数据元素**:
```c
void printList(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
```
6. **逆序显示链表中的数据元素**:
```c
void reversePrintList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n"); // 避免逆序后立即打印导致的数据错位
reverseList(head); // 另外定义一个函数反转链表,然后在此处调用
}
// 反转链表辅助函数
void reverseList(Node** head) {
Node* prev = NULL;
Node* current = *head;
Node* next = NULL;
while (current != NULL) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
*head = prev;
}
```
阅读全文
相关推荐
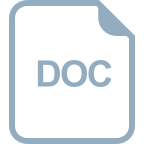
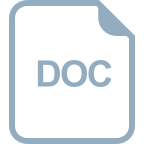
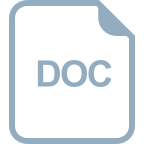















