设计并实现一个平面向量类Vector,包含私有数据成员__x、__y,提供公开方法add()、sub()、mul()实现向量运算,以及show()方法查看向量值。添加只读属性length计算并返回向量长度。编写程序测试类的各项功能。、
时间: 2024-10-18 16:17:37 浏览: 51
设计一个名为`Vector`的平面向量类,我们可以按照以下步骤进行:
首先,创建一个名为`Vector`的类,并包含两个私有数据成员`__x`和`__y`,分别表示向量的水平分量和垂直分量。
```cpp
class Vector {
private:
double __x;
double __y;
public:
// 构造函数
Vector(double x = 0, double y = 0) : __x(x), __y(y) {}
// 只读属性,获取向量长度
double length() const {
return std::sqrt(__x * __x + __y * __y);
}
// 向量加法
Vector add(const Vector& other) const {
return Vector(__x + other.__x, __y + other.__y);
}
// 向量减法
Vector sub(const Vector& other) const {
return Vector(__x - other.__x, __y - other.__y);
}
// 向量乘法(点积)
double mul(const Vector& other) const {
return __x * other.__x + __y * other.__y;
}
// 显示向量值
void show() const {
std::cout << "Vector (" << __x << ", " << __y << ")\n";
}
};
```
接下来,我们编写一个简单的测试程序来验证这个类的功能:
```cpp
int main() {
Vector v1(3, 4); // 创建第一个向量
Vector v2(5, 6); // 创建第二个向量
// 测试加法
Vector result = v1.add(v2);
result.show();
std::cout << "Length of addition: " << result.length() << "\n";
// 测试减法
result = v1.sub(v2);
result.show();
std::cout << "Length of subtraction: " << result.length() << "\n";
// 测试乘法
double dotProduct = v1.mul(v2);
std::cout << "Dot product: " << dotProduct << "\n";
return 0;
}
```
运行这个测试程序,你可以看到向量加法、减法的结果以及它们的长度,还有向量之间的点积。
阅读全文
相关推荐
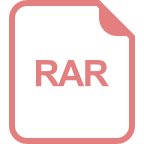
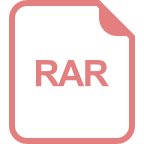
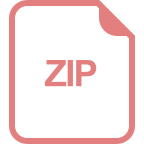




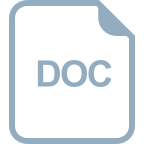
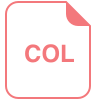
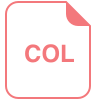
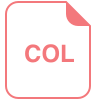
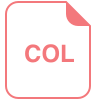
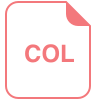

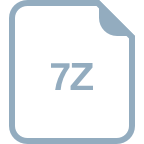
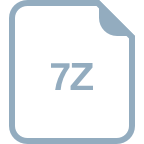
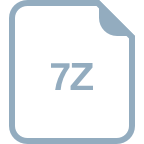
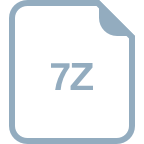
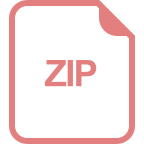