for _ in range(400): # 生成随机坐标 x = random.uniform(0, 1) y = random.uniform(0, 1) # 执行点击操作 d.click(x, y) 不点击指定按钮,怎么写
时间: 2023-08-31 19:07:02 浏览: 83
如果您想在循环中执行随机点击操作而不是点击指定按钮,可以使用random模块生成随机的坐标,并将这些坐标传递给`d.click()`方法来模拟点击。以下是一个示例代码片段:
```python
# 导入Weditor库和random模块
from weditor import Weditor
import random
# 创建Weditor实例
d = Weditor()
# 设置循环次数
click_times = 400
# 循环执行随机点击操作
for _ in range(click_times):
# 生成随机坐标
x = random.uniform(0, 1)
y = random.uniform(0, 1)
# 执行点击操作
d.click(x, y)
```
请注意,以上代码假设您已经正确安装并配置了Weditor库,并已经实例化了Weditor对象(使用"d = Weditor()")。同时,您可以根据实际需求调整循环次数(click_times)。这样,代码将在Weditor中模拟随机点击操作,而不是点击指定按钮。
相关问题
import random import numpy as np import matplotlib.pyplot as plt 生成随机坐标点 def generate_points(num_points): points = [] for i in range(num_points): x = random.uniform(-10, 10) y = random.uniform(-10, 10) points.append([x, y]) return points 计算欧几里得距离 def euclidean_distance(point1, point2): return np.sqrt(np.sum(np.square(np.array(point1) - np.array(point2)))) K-means算法实现 def kmeans(points, k, num_iterations=100): num_points = len(points) # 随机选择k个点作为初始聚类中心 centroids = random.sample(points, k) # 初始化聚类标签和距离 labels = np.zeros(num_points) distances = np.zeros((num_points, k)) for i in range(num_iterations): # 计算每个点到每个聚类中心的距离 for j in range(num_points): for l in range(k): distances[j][l] = euclidean_distance(points[j], centroids[l]) # 根据距离将点分配到最近的聚类中心 for j in range(num_points): labels[j] = np.argmin(distances[j]) # 更新聚类中心 for l in range(k): centroids[l] = np.mean([points[j] for j in range(num_points) if labels[j] == l], axis=0) return labels, centroids 生成坐标点 points = generate_points(100) 对点进行K-means聚类 k_values = [2, 3, 4] for k in k_values: labels, centroids = kmeans(points, k) # 绘制聚类结果 colors = [‘r’, ‘g’, ‘b’, ‘y’, ‘c’, ‘m’] for i in range(k): plt.scatter([points[j][0] for j in range(len(points)) if labels[j] == i], [points[j][1] for j in range(len(points)) if labels[j] == i], color=colors[i]) plt.scatter([centroid[0] for centroid in centroids], [centroid[1] for centroid in centroids], marker=‘x’, color=‘k’, s=100) plt.title(‘K-means clustering with k={}’.format(k)) plt.show()import numpy as np import pandas as pd import matplotlib.pyplot as plt from sklearn.cluster import KMeans from sklearn.datasets import load_iris 载入数据集 iris = load_iris() X = iris.data y = iris.target K-means聚类 kmeans = KMeans(n_clusters=3, random_state=0).fit(X) 可视化结果 plt.scatter(X[:, 0], X[:, 1], c=kmeans.labels_) plt.xlabel(‘Sepal length’) plt.ylabel(‘Sepal width’) plt.title(‘K-means clustering on iris dataset’) plt.show()从聚类算法的评价指标对结果进行分析
常用的聚类算法评价指标有以下几种:
1. SSE(Sum of Squared Errors):簇内误差平方和,即簇内各点到簇中心的距离平方和,SSE越小表示簇内数据越紧密。
2. Silhouette Coefficient(轮廓系数):用于衡量样本聚类的密集程度,取值范围在[-1,1]之间,越接近1表示聚类效果越好。
3. Calinski-Harabasz Index(方差比率准则):通过计算类间离散度与类内离散度的比值来评估聚类的效果,CHI越大表示聚类效果越好。
4. Davies-Bouldin Index(DBI):通过计算簇内的平均距离和簇间的距离来衡量聚类效果,DBI越小表示聚类效果越好。
对于以上评价指标,可以根据具体的业务场景和需求选择合适的指标进行评估。在实际使用中,可以采用交叉验证等方法来验证聚类算法的效果。
import random n = 1000000 # 抽样次数 count = 0 # 落入圆内的点数 for i in range(n): x, y = random.uniform(-1, 1), random.uniform(-1, 1) if x**2 + y**2 <= 1: count += 1 pi = 4 * count / n # 估算出的圆周率 print(pi)
这段代码是一个基于Python的蒙特卡洛仿真的示例,用于估算圆周率。具体实现过程如下:
1. 首先导入了random库,用于生成随机数。
2. 设置了抽样次数n和计数器count。
3. 使用for循环进行抽样。每次循环中,使用random.uniform函数生成两个在-1到1之间的随机数x和y,表示一个点的坐标。如果该点在圆内,则将计数器count加1。
4. 循环结束后,根据公式pi=4*count/n计算估算出的圆周率,并打印输出。
需要注意的是,蒙特卡洛仿真的结果存在一定的误差,其精度与抽样次数和随机数生成算法有关。
相关推荐
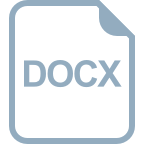
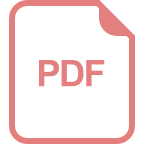












