创建一条管道,实现两个子进程之间的通信,实现并给出代码
时间: 2024-11-20 08:43:58 浏览: 26
创建管道在Unix/Linux系统中是一种常见的用于进程间通信(IPC,Inter-Process Communication)的方式。管道允许一个进程将数据发送给另一个正在等待接收的进程,通常通过`pipe()`函数在C语言中创建。这里是一个简单的示例,展示了如何在Linux环境下使用管道创建两个子进程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#define BUFFER_SIZE 50
int main() {
// 创建管道
int pipefd[2];
if (pipe(pipefd) == -1) {
perror("Failed to create pipe");
exit(EXIT_FAILURE);
}
pid_t child1_pid, child2_pid;
// 子进程1,读端
if ((child1_pid = fork()) == 0) {
close(pipefd[1]); // 父进程关闭写端
char message[BUFFER_SIZE] = "Hello from Parent";
write(pipefd[0], message, strlen(message) + 1); // 写入管道
printf("Child 1 wrote to pipe and exited.\n");
_exit(0);
} else if (child1_pid > 0) { // 父进程
close(pipefd[0]); // 子进程关闭读端
// 子进程2,写端
pid_t child2_pid = fork();
if (child2_pid == 0) {
char buffer[BUFFER_SIZE];
read(pipefd[1], buffer, BUFFER_SIZE); // 从管道读取
printf("Child 2 received: %s\n", buffer);
_exit(0);
}
wait(NULL); // 等待子进程结束
} else {
perror("Fork failed");
exit(EXIT_FAILURE);
}
return 0;
}
```
在这个例子中,父进程首先创建管道,然后分别fork出两个子进程。子进程1负责向管道写入消息,而子进程2负责读取。当所有操作完成后,主进程退出。
阅读全文
相关推荐
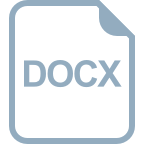
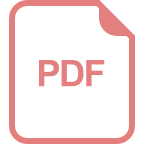
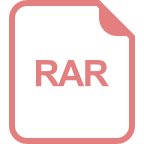



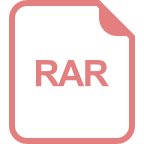








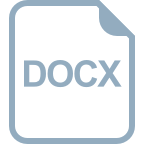
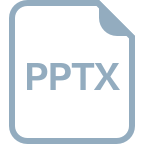
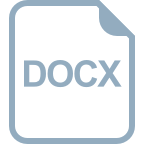