python画多个折线图
时间: 2024-09-24 15:18:27 浏览: 30
在Python中,我们可以使用matplotlib库来绘制多个折线图。以下是基本步骤:
1. 首先,你需要安装matplotlib,如果还没有安装可以使用pip install matplotlib命令。
2. 导入所需的模块:
```python
import matplotlib.pyplot as plt
```
3. 准备数据,这里假设你有两个列表,每个列表对应一条折线的数据:
```python
data1 = [1, 4, 9, 16, 25] # 第一条线的数据
data2 = [2, 5, 8, 11, 14] # 第二条线的数据
```
4. 使用`plt.plot()`函数绘制两条折线,并给它们指定标签,方便区分:
```python
plt.plot(data1, label='直线A')
plt.plot(data2, label='直线B')
```
5. 添加标题、轴标签以及图例:
```python
plt.title('两个折线图示例')
plt.xlabel('X轴')
plt.ylabel('Y轴')
plt.legend() # 显示图例
```
6. 最后,使用`plt.show()`显示图形:
```python
plt.show()
```
如果你想在同一张图上添加更多折线,只需多次调用`plt.plot()`并添加对应的标签即可。
相关问题
python画多个折线图颜色透明度如何设置
在Python中,绘制多个折线图并设置颜色透明度,通常会使用matplotlib库来进行。以下是一个简单的示例代码,展示如何为多个折线图设置颜色透明度:
```python
import matplotlib.pyplot as plt
import numpy as np
# 假设有两组数据
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
# 设置每个折线图的颜色和透明度
colors = ['red', 'green'] # 折线图的颜色
alphas = [0.5, 0.8] # 对应的颜色透明度,取值范围是0到1
# 创建一个图形
plt.figure()
# 绘制折线图并设置颜色和透明度
for i in range(len(y1)):
plt.plot(x, y1[i], color=colors[i % len(colors)], alpha=alphas[i % len(alphas)], label='sin' if i == 0 else '')
for i in range(len(y2)):
plt.plot(x, y2[i], color=colors[i % len(colors)], alpha=alphas[i % len(alphas)], label='cos' if i == 0 else '')
# 添加图例
plt.legend()
# 显示图形
plt.show()
```
在这段代码中,我们首先导入了matplotlib.pyplot和numpy库。然后创建了两组数据,分别代表sin和cos函数的值。接着定义了两个列表,一个用于指定颜色,另一个用于指定透明度。在绘制每条折线时,通过`color`参数设置颜色,通过`alpha`参数设置透明度。
python画多个折线图,横坐标为文字,折线图用不同颜色,不同现型表示
可以使用 matplotlib 库来画多个折线图,具体步骤如下:
1. 导入 matplotlib 库和 numpy 库:
```python
import matplotlib.pyplot as plt
import numpy as np
```
2. 准备数据,将横坐标和纵坐标分别存储在两个数组中:
```python
x = np.array(['A', 'B', 'C', 'D', 'E'])
y1 = np.array([1, 3, 2, 4, 5])
y2 = np.array([3, 2, 4, 1, 6])
y3 = np.array([2, 4, 3, 5, 1])
```
3. 使用 plot 函数画折线图,设置不同的颜色和线型:
```python
plt.plot(x, y1, color='red', linestyle='solid', label='Line 1')
plt.plot(x, y2, color='green', linestyle='dashed', label='Line 2')
plt.plot(x, y3, color='blue', linestyle='dashdot', label='Line 3')
```
4. 添加图例和标签:
```python
plt.legend()
plt.xlabel('X Label')
plt.ylabel('Y Label')
```
5. 显示图形:
```python
plt.show()
```
这样就可以画出多个折线图,横坐标为文字,折线图用不同颜色、不同线型表示。
相关推荐
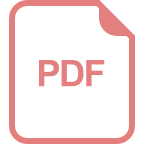
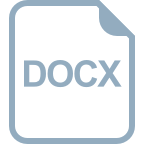
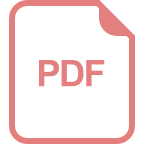












