用C++做俄罗斯方块游戏
时间: 2024-06-13 11:09:08 浏览: 12
以下是用C++实现俄罗斯方块游戏的基本步骤:
1.定义方块的形状和颜色,可以使用二维数组来表示方块的形状,使用枚举类型来表示颜色。
2.定义游戏区域,可以使用二维数组来表示游戏区域,其中0表示该位置为空,1表示该位置有方块。
3.定义方块的移动和旋转,可以使用函数来实现方块的移动和旋转,例如moveLeft()、moveRight()、rotate()等函数。
4.定义方块的下落,可以使用定时器来实现方块的下落,每隔一定时间就调用一次下落函数。
5.定义方块的消除,当一行被填满时,就将该行消除,并将上面的方块下落。
6.定义游戏结束的条件,当方块无法下落时,游戏结束。
以下是一个简单的C++俄罗斯方块游戏的代码示例:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
const int ROWS = 20;
const int COLS = 10;
enum Color { BLACK, RED, GREEN, YELLOW, BLUE, MAGENTA, CYAN, WHITE };
int board[ROWS][COLS] = { 0 };
int shapes[7][4][4] = {
{
{ 0, 0, 0, 0 },
{ 1, 1, 1, 1 },
{ 0, 0, 0, 0 },
{ 0, 0, 0, 0 }
},
{
{ 0, 0, 0, 0 },
{ 0, 2, 2, 0 },
{ 0, 2, 2, 0 },
{ 0, 0, 0, 0 }
},
{
{ 0, 0, 0, 0 },
{ 0, 3, 0, 0 },
{ 3, 3, 3, 0 },
{ 0, 0, 0, 0 }
},
{
{ 0, 0, 0, 0 },
{ 0, 0, 4, 4 },
{ 0, 4, 4, 0 },
{ 0, 0, 0, 0 }
},
{
{ 0, 0, 0, 0 },
{ 0, 5, 5, 0 },
{ 5, 5, 0, 0 },
{ 0, 0, 0, 0 }
},
{
{ 0, 0, 0, 0 },
{ 6, 6, 0, 0 },
{ 0, 6, 6, 0 },
{ 0, 0, 0, 0 }
},
{
{ 0, 0, 0, 0 },
{ 0, 7, 0, 0 },
{ 7, 7, 7, 0 },
{ 0, 0, 0, 0 }
}
};
Color colors[8] = { BLACK, RED, GREEN, YELLOW, BLUE, MAGENTA, CYAN, WHITE };
struct Point {
int x;
int y;
};
class Tetris {
public:
Tetris();
void run();
private:
void initBoard();
void drawBoard();
void drawShape(int x, int y, int shape);
void eraseShape(int x, int y, int shape);
bool isOverlap(int x, int y, int shape);
void addShape(int x, int y, int shape);
void removeFullLines();
bool isGameOver();
void newShape();
void moveLeft();
void moveRight();
void rotate();
void moveDown();
void drop();
void clearScreen();
void setColor(Color color);
void gotoxy(int x, int y);
int getRandomShape();
Point current;
int currentShape;
int score;
};
Tetris::Tetris() {
srand(time(NULL));
initBoard();
score = 0;
}
void Tetris::run() {
newShape();
while (!isGameOver()) {
clearScreen();
drawBoard();
moveDown();
removeFullLines();
gotoxy(0, ROWS + 2);
cout << "Score: " << score << endl;
cout << "Press any key to continue..." << endl;
cin.get();
}
clearScreen();
gotoxy(0, ROWS / 2);
cout << "Game Over!" << endl;
}
void Tetris::initBoard() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
board[i][j] = 0;
}
}
}
void Tetris::drawBoard() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j] != 0) {
setColor((Color)board[i][j]);
drawShape(j * 2, i, board[i][j]);
}
}
}
setColor(WHITE);
}
void Tetris::drawShape(int x, int y, int shape) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (shapes[shape][i][j] != 0) {
gotoxy(x + j * 2, y + i);
cout << "[]";
}
}
}
}
void Tetris::eraseShape(int x, int y, int shape) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (shapes[shape][i][j] != 0) {
gotoxy(x + j * 2, y + i);
cout << " ";
}
}
}
}
bool Tetris::isOverlap(int x, int y, int shape) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (shapes[shape][i][j] != 0) {
int row = y + i;
int col = x + j;
if (row < 0 || row >= ROWS || col < 0 || col >= COLS || board[row][col] != 0) {
return true;
}
}
}
}
return false;
}
void Tetris::addShape(int x, int y, int shape) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (shapes[shape][i][j] != 0) {
int row = y + i;
int col = x + j;
board[row][col] = shape + 1;
}
}
}
}
void Tetris::removeFullLines() {
int count = 0;
for (int i = ROWS - 1; i >= 0; i--) {
bool full = true;
for (int j = 0; j < COLS; j++) {
if (board[i][j] == 0) {
full = false;
break;
}
}
if (full) {
count++;
for (int k = i; k > 0; k--) {
for (int j = 0; j < COLS; j++) {
board[k][j] = board[k - 1][j];
}
}
for (int j = 0; j < COLS; j++) {
board[0][j] = 0;
}
i++;
}
}
score += count * count;
}
bool Tetris::isGameOver() {
return isOverlap(current.x, current.y, currentShape);
}
void Tetris::newShape() {
current.x = COLS / 2 - 2;
current.y = 0;
currentShape = getRandomShape();
if (isOverlap(current.x, current.y, currentShape)) {
score = 0;
initBoard();
}
addShape(current.x, current.y, currentShape);
}
void Tetris::moveLeft() {
eraseShape(current.x, current.y, currentShape);
if (!isOverlap(current.x - 1, current.y, currentShape)) {
current.x--;
}
addShape(current.x, current.y, currentShape);
}
void Tetris::moveRight() {
eraseShape(current.x, current.y, currentShape);
if (!isOverlap(current.x + 1, current.y, currentShape)) {
current.x++;
}
addShape(current.x, current.y, currentShape);
}
void Tetris::rotate() {
eraseShape(current.x, current.y, currentShape);
int tempShape = currentShape;
currentShape = (currentShape + 1) % 4;
if (isOverlap(current.x, current.y, currentShape)) {
currentShape = tempShape;
}
addShape(current.x, current.y, currentShape);
}
void Tetris::moveDown() {
eraseShape(current.x, current.y, currentShape);
if (!isOverlap(current.x, current.y + 1, currentShape)) {
current.y++;
}
else {
addShape(current.x, current.y, currentShape);
newShape();
}
addShape(current.x, current.y, currentShape);
}
void Tetris::drop() {
eraseShape(current.x, current.y, currentShape);
while (!isOverlap(current.x, current.y + 1, currentShape)) {
current.y++;
}
addShape(current.x, current.y, currentShape);
newShape();
}
void Tetris::clearScreen() {
system("cls");
}
void Tetris::setColor(Color color) {
switch (color) {
case BLACK:
cout << "\033[30m";
break;
case RED:
cout << "\033[31m";
break;
case GREEN:
cout << "\033[32m";
break;
case YELLOW:
cout << "\033[33m";
break;
case BLUE:
cout << "\033[34m";
break;
case MAGENTA:
cout << "\033[35m";
break;
case CYAN:
cout << "\033[36m";
break;
case WHITE:
cout << "\033[37m";
break;
}
}
void Tetris::gotoxy(int x, int y) {
printf("\033[%d;%dH", y + 1, x + 1);
}
int Tetris::getRandomShape() {
return rand() % 7;
}
int main() {
Tetris tetris;
tetris.run();
return 0;
}
```
相关推荐
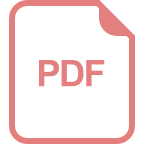














