写c++制作俄罗斯方块游戏代码
时间: 2023-04-03 10:04:55 浏览: 114
感谢您的提问。以下是制作俄罗斯方块游戏的 C 代码示例:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 10
#define HEIGHT 20
int board[HEIGHT][WIDTH] = {0};
int shapes[7][4][2] = {
{{0, 0}, {0, 1}, {1, 0}, {1, 1}}, // O
{{0, 0}, {0, 1}, {0, 2}, {0, 3}}, // I
{{0, 0}, {0, 1}, {0, 2}, {1, 2}}, // L
{{0, 0}, {0, 1}, {0, 2}, {-1, 2}}, // J
{{0, 0}, {0, 1}, {1, 1}, {1, 2}}, // S
{{0, 0}, {0, 1}, {-1, 1}, {1, 0}}, // Z
{{0, 0}, {0, 1}, {1, 1}, {0, 2}} // T
};
int current_shape = 0;
int current_rotation = 0;
int current_x = 0;
int current_y = 0;
void draw_board() {
system("cls");
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (board[i][j] == 0) {
printf(".");
} else {
printf("*");
}
}
printf("\n");
}
}
void draw_shape(int shape, int rotation, int x, int y) {
for (int i = 0; i < 4; i++) {
int row = y + shapes[shape][i][0];
int col = x + shapes[shape][i][1];
board[row][col] = 1;
}
}
void erase_shape(int shape, int rotation, int x, int y) {
for (int i = 0; i < 4; i++) {
int row = y + shapes[shape][i][0];
int col = x + shapes[shape][i][1];
board[row][col] = 0;
}
}
int check_collision(int shape, int rotation, int x, int y) {
for (int i = 0; i < 4; i++) {
int row = y + shapes[shape][i][0];
int col = x + shapes[shape][i][1];
if (row < 0 || row >= HEIGHT || col < 0 || col >= WIDTH || board[row][col] == 1) {
return 1;
}
}
return 0;
}
void spawn_shape() {
current_shape = rand() % 7;
current_rotation = rand() % 4;
current_x = WIDTH / 2 - 1;
current_y = 0;
if (check_collision(current_shape, current_rotation, current_x, current_y)) {
printf("Game over!\n");
exit(0);
}
draw_shape(current_shape, current_rotation, current_x, current_y);
}
void rotate_shape() {
erase_shape(current_shape, current_rotation, current_x, current_y);
current_rotation = (current_rotation + 1) % 4;
if (check_collision(current_shape, current_rotation, current_x, current_y)) {
current_rotation = (current_rotation - 1 + 4) % 4;
}
draw_shape(current_shape, current_rotation, current_x, current_y);
}
void move_shape(int dx, int dy) {
erase_shape(current_shape, current_rotation, current_x, current_y);
current_x += dx;
current_y += dy;
if (check_collision(current_shape, current_rotation, current_x, current_y)) {
current_x -= dx;
current_y -= dy;
}
draw_shape(current_shape, current_rotation, current_x, current_y);
}
void drop_shape() {
while (!check_collision(current_shape, current_rotation, current_x, current_y + 1)) {
move_shape(0, 1);
}
}
void clear_lines() {
int lines_cleared = 0;
for (int i = HEIGHT - 1; i >= 0; i--) {
int line_full = 1;
for (int j = 0; j < WIDTH; j++) {
if (board[i][j] == 0) {
line_full = 0;
break;
}
}
if (line_full) {
lines_cleared++;
for (int k = i; k > 0; k--) {
for (int j = 0; j < WIDTH; j++) {
board[k][j] = board[k - 1][j];
}
}
for (int j = 0; j < WIDTH; j++) {
board[0][j] = 0;
}
i++;
}
}
if (lines_cleared > 0) {
printf("Lines cleared: %d\n", lines_cleared);
Sleep(1000);
}
}
int main() {
srand(time(NULL));
spawn_shape();
while (1) {
if (_kbhit()) {
int key = _getch();
switch (key) {
case 'a':
move_shape(-1, 0);
break;
case 'd':
move_shape(1, 0);
break;
case 's':
move_shape(0, 1);
break;
case 'w':
rotate_shape();
break;
case ' ':
drop_shape();
break;
}
}
move_shape(0, 1);
clear_lines();
draw_board();
Sleep(100);
}
return 0;
}
相关推荐
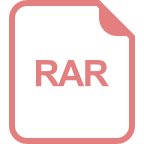















