在Andriod studio 上设计ExpandableListView
时间: 2024-04-18 09:07:20 浏览: 6
1. 创建一个新的Android项目,选择Empty Activity。
2. 在MainActivity中添加ExpandableListView控件,设置其layout_width和layout_height为match_parent。
3. 在res/layout文件夹中创建一个新的xml文件,命名为list_group.xml。在该文件中添加一个TextView控件,设置其layout_width和layout_height为match_parent,并设置其背景颜色和字体颜色。
4. 在res/layout文件夹中创建另一个新的xml文件,命名为list_item.xml。在该文件中添加一个TextView控件,设置其layout_width和layout_height为match_parent,并设置其背景颜色和字体颜色。
5. 在MainActivity中创建一个ExpandableListAdapter类,该类继承自BaseExpandableListAdapter,并实现其方法。
6. 在MainActivity的onCreate方法中,创建数据源并设置ExpandableListView的适配器为ExpandableListAdapter。
7. 运行程序,查看ExpandableListView效果。
下面是一个示例代码:
```
public class MainActivity extends AppCompatActivity {
ExpandableListView expandableListView;
ExpandableListAdapter expandableListAdapter;
List<String> groupList;
List<String> childList;
Map<String, List<String>> itemMap;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
expandableListView = findViewById(R.id.expandableListView);
initData();
expandableListAdapter = new ExpandableListAdapter(this, groupList, itemMap);
expandableListView.setAdapter(expandableListAdapter);
}
private void initData() {
groupList = new ArrayList<>();
childList = new ArrayList<>();
itemMap = new HashMap<>();
groupList.add("Group 1");
groupList.add("Group 2");
groupList.add("Group 3");
childList.add("Item 1");
childList.add("Item 2");
childList.add("Item 3");
childList.add("Item 4");
childList.add("Item 5");
itemMap.put(groupList.get(0), childList);
itemMap.put(groupList.get(1), childList);
itemMap.put(groupList.get(2), childList);
}
}
```
ExpandableListAdapter类代码:
```
public class ExpandableListAdapter extends BaseExpandableListAdapter {
private Context context;
private List<String> groupList;
private Map<String, List<String>> itemMap;
public ExpandableListAdapter(Context context, List<String> groupList, Map<String, List<String>> itemMap) {
this.context = context;
this.groupList = groupList;
this.itemMap = itemMap;
}
@Override
public int getGroupCount() {
return groupList.size();
}
@Override
public int getChildrenCount(int groupPosition) {
return itemMap.get(groupList.get(groupPosition)).size();
}
@Override
public Object getGroup(int groupPosition) {
return groupList.get(groupPosition);
}
@Override
public Object getChild(int groupPosition, int childPosition) {
return itemMap.get(groupList.get(groupPosition)).get(childPosition);
}
@Override
public long getGroupId(int groupPosition) {
return groupPosition;
}
@Override
public long getChildId(int groupPosition, int childPosition) {
return childPosition;
}
@Override
public boolean hasStableIds() {
return false;
}
@Override
public View getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) {
if (convertView == null) {
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
convertView = inflater.inflate(R.layout.list_group, null);
}
TextView textView = convertView.findViewById(R.id.textView);
textView.setText(groupList.get(groupPosition));
return convertView;
}
@Override
public View getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertView, ViewGroup parent) {
if (convertView == null) {
LayoutInflater inflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
convertView = inflater.inflate(R.layout.list_item, null);
}
TextView textView = convertView.findViewById(R.id.textView);
textView.setText(itemMap.get(groupList.get(groupPosition)).get(childPosition));
return convertView;
}
@Override
public boolean isChildSelectable(int groupPosition, int childPosition) {
return true;
}
}
```
相关推荐
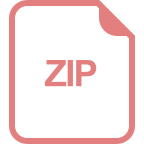














