unity coroutine
时间: 2023-12-22 19:01:41 浏览: 108
Unity coroutine 是一种在Unity游戏引擎中使用的协程功能。协程允许在程序执行时暂停和继续执行特定的代码片段,从而能够实现异步操作和延迟执行。
通过使用协程,我们可以在游戏中实现很多有用的功能,比如延迟执行某个代码块,逐帧更新一个复杂的操作,或者在一段时间内执行某个任务。相比于传统的线程或定时器,协程更加轻量级且易于管理,能够更好地适应游戏开发中的需求。
在Unity中使用协程非常简单,只需要在对应的方法前面加上关键字" IEnumerator",然后在方法体中使用"yield return"来暂停执行或者继续执行。通过这种方式,我们可以编写出清晰、可读性高的异步代码,而不必担心复杂的线程管理和同步问题。
总的来说,Unity协程是一种强大的工具,能够帮助我们实现复杂的异步操作和操作流程控制,同时也能够提高代码的可维护性和可读性。借助协程,我们能够更加高效地开发出高质量、流畅的游戏。
相关问题
Unity Coroutine continue failure
Unity中的Coroutine是一种轻量级的任务调度机制,用于异步执行游戏循环中的任务序列。当你尝试继续一个已经中断(`Stop()` 或 `Cancel()`)的Coroutine时,可能会遇到"continue failure",这通常表示该Coroutine的状态已不再是可继续的。
当Coroutine在运行过程中被停止或取消,它的内部状态就被设置成了停止状态,这时试图通过`yield return null`或者`yield break`继续它将导致失败。如果你尝试恢复这样的Coroutine,通常需要重新创建一个新的Coroutine实例,并从上一次暂停的地方开始。
例如:
```csharp
IEnumerator MyCoroutine()
{
while (true)
{
yield return new WaitForSeconds(0.5f);
// 如果在这个位置被stop或cancel
if (SomeCondition) // 假设条件变为false,我们停止了
StopCoroutine(MyCoroutine()); // 这里会抛出continue failure
// 下面这部分代码无法执行,因为Coroutine已被停止
// ...
}
}
// 使用时
StartCoroutine(MyCoroutine());
```
为了避免这种错误,你应该在`MyCoroutine`中处理异常情况时,提前检查是否可以继续,或者在外部有一个标志控制Coroutine的生命周期。
unity Coroutine couldn't be started because the the game object 'ModelsScene' is i
This error message in Unity usually occurs when you try to start a coroutine on a GameObject that is inactive or has been destroyed.
To fix this issue, you should first check if the GameObject 'ModelsScene' is active and not destroyed. If it is destroyed or inactive, you can use the `GameObject.Find` method to get a reference to a new instance of the object, if it exists.
Here's an example of how you can modify your code to handle this error:
```
public GameObject modelsScene;
void Start()
{
StartCoroutine(MyCoroutine());
}
IEnumerator MyCoroutine()
{
// Check if the modelsScene object exists and is active
if (modelsScene != null && modelsScene.activeInHierarchy)
{
// Your coroutine code here
yield return new WaitForSeconds(2.0f);
}
else
{
Debug.LogError("The modelsScene object is invalid or inactive.");
}
}
```
By checking if the `modelsScene` object is valid and active before starting the coroutine, you can avoid the error message you were experiencing.
阅读全文
相关推荐
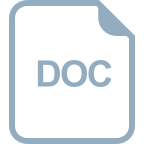
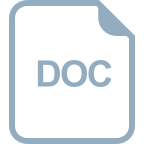
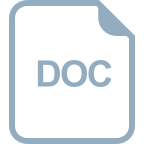
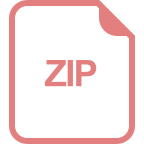
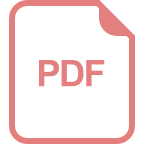
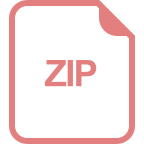
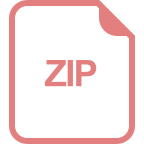


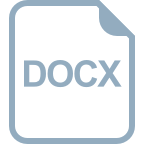
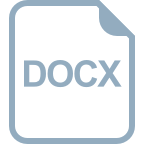
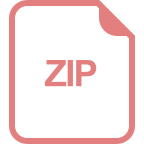
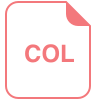
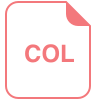


