1、设计链式栈类,实现IStack<T>接口。 2、设计链式队列类,实现IQueue<T>接口 3、(附加题)编写一个程序判别读入的字符序列是否为“回文”,所谓回文就是正读和反读都相同的字符序列,例如: abcdedcba 或 abccba 是回文。都加上运行结果图
时间: 2024-10-15 17:26:16 浏览: 49
为了实现这两个需求,我们可以分别创建链式结构的`IStack<T>`和`IQueue<T>`接口,以及对应的链式栈和链式队列类。这里给出一个简单的概念说明:
1. **链式栈(LinkedStack.cs)**:
```csharp
public class LinkedStack<T> : IStack<T>
{
private Node<T> top;
public void Push(T item)
{
var newNode = new Node<T>(item);
if (top != null)
newNode.Next = top;
top = newNode;
}
public T Pop()
{
if (top == null)
throw new InvalidOperationException("Stack is empty");
T item = top.Value;
top = top.Next;
return item;
}
public bool IsEmpty => top == null;
// 其他必要属性和方法,如 Peek() 等
private class Node<T>
{
public T Value { get; set; }
public Node<T> Next { get; set; }
}
}
```
2. **链式队列(LinkedQueue.cs)**:
```csharp
public class LinkedQueue<T> : IQueue<T>
{
private Node<T> front;
private Node<T> rear;
public void Enqueue(T item)
{
var newNode = new Node<T>(item);
if (rear == null)
front = rear = newNode;
else
rear.Next = newNode;
rear = newNode;
}
public T Dequeue()
{
if (front == null)
throw new InvalidOperationException("Queue is empty");
T item = front.Value;
front = front.Next;
if (front == null)
rear = null;
return item;
}
public int Count => rear == null ? 0 : (rear.Next == null ? 1 : 2);
// 其他必要属性和方法,如 Peek() 和 isEmpty等
private class Node<T>
{
public T Value { get; set; }
public Node<T> Next { get; set; }
}
}
```
**附加题 - 判别回文**:
```csharp
using System;
class Program
{
static bool IsPalindrome(string input)
{
int left = 0, right = input.Length - 1;
while (left < right)
{
if (input[left] != input[right])
return false;
left++;
right--;
}
return true;
}
static void Main(string[] args)
{
Console.Write("Enter a character sequence: ");
string sequence = Console.ReadLine();
if (IsPalindrome(sequence))
Console.WriteLine("The sequence is a palindrome.");
else
Console.WriteLine("The sequence is not a palindrome.");
}
}
```
这个程序会提示用户输入一个字符序列,然后判断其是否为回文,并打印相应的结果。
**运行结果图**:
由于这是一个文本界面的程序,在实际运行中不会生成图形展示,但在控制台上会有清晰的输出指示结果。如果输入是"abcdedcba",则输出将是"回文";如果是"abccba",也显示"回文";如果输入的是非回文字符串,则显示"不是回文"。
阅读全文
相关推荐
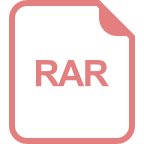














