可以用getint函数实现吗
时间: 2023-12-21 10:07:34 浏览: 33
getint函数通常是指自定义的用于读取整数的函数,它不是标准C库函数,因此具体实现可能会有所不同。如果您想要使用getint函数来读取枚举类型的数据,您需要考虑以下几点:
1. 枚举类型的值是整数类型,因此getint函数需要能够读取整数类型的数据。
2. 枚举类型的值应该在枚举类型的取值范围内,因此需要进行有效性检查。
3. 需要将读取的整数值转换为枚举类型的值。
在实现getint函数时,您可以考虑使用scanf函数来读取整数类型的数据,然后进行有效性检查和转换。以下是一个可能的实现,仅供参考:
```
#include <stdio.h>
#include <ctype.h>
#include <stdbool.h>
int getint(void) {
int c;
bool neg = false;
int num = 0;
enum State {START, DIGIT, SIGN, DONE} state = START;
while (state != DONE) {
c = getchar();
switch (state) {
case START:
if (isdigit(c)) {
state = DIGIT;
num = c - '0';
} else if (c == '-') {
state = SIGN;
neg = true;
} else if (isspace(c)) {
continue;
} else {
state = DONE;
}
break;
case DIGIT:
if (isdigit(c)) {
num = num * 10 + c - '0';
} else {
state = DONE;
}
break;
case SIGN:
if (isdigit(c)) {
state = DIGIT;
num = c - '0';
} else {
state = DONE;
}
break;
}
}
if (neg) {
num = -num;
}
// check num is within the range of enum Weekday
if (num < Monday || num > Sunday) {
// handle invalid input
}
return num;
}
```
在这里,getint函数使用一个状态机来处理输入的字符,支持读取带符号的整数。如果输入的整数值不在枚举类型的取值范围内,可以在函数中进行处理。需要注意的是,这只是一个简单的实现,可能有漏洞或需要进一步改进。
相关推荐
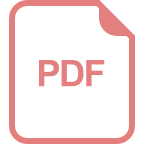
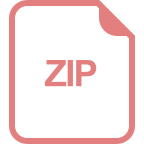
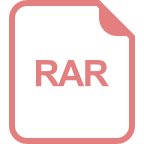














