用C语言完成以下程序:现有三个进程分别问进程一,进程二和进程三,两个管道,管道一和管道二,进程一负责从data.txt中读取数值并放入管道一中,注意,data.txt中存放的都是整型数据;进程二从管道中取走数据,对每一个数据进行加1的操作,并将所有新的数据放入管道二中;进程三负责从管道三中取出数据并将其存放在result.txt文件中。注意,result.txt文件一开始并不存在。最后,程序结束。请给C语言代码。
时间: 2024-03-26 18:34:19 浏览: 44
以下是题目所需的C语言代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
#include <fcntl.h>
#define PIPE1_READ 0
#define PIPE1_WRITE 1
#define PIPE2_READ 2
#define PIPE2_WRITE 3
int main() {
int pipe1[2], pipe2[2];
pid_t pid1, pid2, pid3;
if (pipe(pipe1) < 0 || pipe(pipe2) < 0) {
perror("pipe error");
exit(EXIT_FAILURE);
}
if ((pid1 = fork()) < 0) {
perror("fork error");
exit(EXIT_FAILURE);
} else if (pid1 == 0) {
close(pipe1[PIPE_READ]);
close(pipe2[PIPE_READ]);
close(pipe2[PIPE_WRITE]);
int fd = open("data.txt", O_RDONLY);
if (fd < 0) {
perror("open error");
exit(EXIT_FAILURE);
}
int num;
while (read(fd, &num, sizeof(num)) > 0) {
write(pipe1[PIPE_WRITE], &num, sizeof(num));
}
close(fd);
close(pipe1[PIPE_WRITE]);
exit(EXIT_SUCCESS);
}
if ((pid2 = fork()) < 0) {
perror("fork error");
exit(EXIT_FAILURE);
} else if (pid2 == 0) {
close(pipe1[PIPE_WRITE]);
close(pipe2[PIPE_READ]);
int num;
while (read(pipe1[PIPE_READ], &num, sizeof(num)) > 0) {
num += 1;
write(pipe2[PIPE_WRITE], &num, sizeof(num));
}
close(pipe1[PIPE_READ]);
close(pipe2[PIPE_WRITE]);
exit(EXIT_SUCCESS);
}
if ((pid3 = fork()) < 0) {
perror("fork error");
exit(EXIT_FAILURE);
} else if (pid3 == 0) {
close(pipe1[PIPE_READ]);
close(pipe1[PIPE_WRITE]);
close(pipe2[PIPE_WRITE]);
int fd = open("result.txt", O_CREAT | O_TRUNC | O_WRONLY, 0644);
if (fd < 0) {
perror("open error");
exit(EXIT_FAILURE);
}
int num;
while (read(pipe2[PIPE_READ], &num, sizeof(num)) > 0) {
write(fd, &num, sizeof(num));
}
close(fd);
close(pipe2[PIPE_READ]);
exit(EXIT_SUCCESS);
}
close(pipe1[PIPE_READ]);
close(pipe1[PIPE_WRITE]);
close(pipe2[PIPE_READ]);
close(pipe2[PIPE_WRITE]);
waitpid(pid1, NULL, 0);
waitpid(pid2, NULL, 0);
waitpid(pid3, NULL, 0);
return 0;
}
```
注意,以上代码仅供参考,可能存在一些错误或不足之处,需要根据实际情况进行修改。
阅读全文
相关推荐
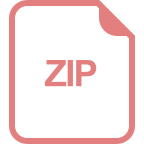





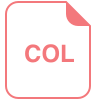


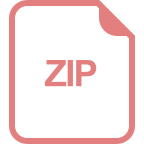
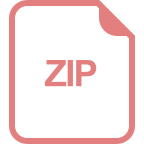
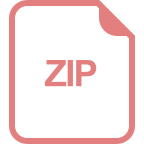
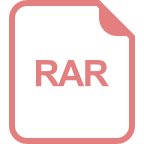