#include <iostream>#include <cmath>using namespace std;class Circle {private: double radius;public: Circle(double r = 0) : radius(r) {} double GetArea() { return M_PI * radius * radius; } double GetPerimeter() { return 2 * M_PI * radius; } void SetRadius(double r) { radius = r; }};class Cone : public Circle {private: double height;public: Cone(double r = 0, double h = 0) : Circle(r), height(h) {} void SetParameter(double r, double h) { SetRadius(r); height = h; } double GetSurfaceArea() { double l = sqrt(height * height + radius * radius); return M_PI * radius * l + M_PI * radius * radius; }};int main() { Cone c(2.5, 5.5); cout << "The surface area of the cone is " << fixed << setprecision(2) << c.GetSurfaceArea() << endl; c.SetParameter(5.5, 6.6); cout << "The surface area of the cone is " << fixed << setprecision(2) << c.GetSurfaceArea() << endl; return 0;}
时间: 2024-04-04 13:31:11 浏览: 12
这段代码实现了一个圆锥体类和一个圆类,并且圆锥体类公有继承了圆类。圆类具有私有数据成员半径(radius)和计算面积(GetArea)、计算周长(GetPerimeter)、修改半径(SetRadius)的成员函数;圆锥体类具有私有数据成员高(height)和计算圆锥体表面积(GetSurfaceArea)、修改圆锥体底面半径和高(SetParameter)的成员函数。在主函数中,定义了一个圆锥体类对象,并初始化底面半径和高分别为2.5和5.5,然后输出圆锥体的表面积。接着,修改圆柱体的底面半径和高分别为5.5和6.6,再次输出圆锥体的表面积。
这段代码的实现比较简单,没有什么问题。不过需要注意的是,圆锥体的表面积计算公式中,开平方根的函数是sqrt,需要在头文件cmath中引入。同时,输出结果需要保留两位小数,可以使用头文件iomanip中的fixed和setprecision函数。
相关问题
c++编程代码差错:#include <iostream>#include <cmath>using namespace std;class Point {public: Point(double x, double y) : x(x), y(y) {} virtual ~Point() {} double getX() const { return x; } double getY() const { return y; }private: double x; double y;};class Circle : public Point {public: Circle(double x, double y, double radius) : Point(x, y), radius(radius) {} virtual ~Circle() {} double getRadius() const { return radius; } double getArea() const { return M_PI * radius * radius; }private: double radius;};int main() { Circle c(1.0, 2.0, 3.0); cout << "Circle with center (" << c.getX() << ", " << c.getY() << ") and radius " << c.getRadius() << endl; cout << "Area: " << c.getArea() << endl; return 0;}
这段代码存在一个错误,即 Circle 类继承了 Point 类,但是我们知道 Circle 不应该是 Point 的子类,因为 Circle 拥有的属性和方法 Point 并没有。正确的做法是使用组合,即在 Circle 类中包含一个 Point 类型的对象。
以下是修改后的代码:
```
#include <iostream>
#include <cmath>
using namespace std;
class Point {
public:
Point(double x, double y) : x(x), y(y) {}
virtual ~Point() {}
double getX() const { return x; }
double getY() const { return y; }
private:
double x;
double y;
};
class Circle {
public:
Circle(double x, double y, double radius) : center(x, y), radius(radius) {}
virtual ~Circle() {}
double getRadius() const { return radius; }
double getArea() const { return M_PI * radius * radius; }
Point getCenter() const { return center; }
private:
Point center;
double radius;
};
int main() {
Circle c(1.0, 2.0, 3.0);
cout << "Circle with center (" << c.getCenter().getX() << ", " << c.getCenter().getY() << ") and radius " << c.getRadius() << endl;
cout << "Area: " << c.getArea() << endl;
return 0;
}
```
修改后的代码使用了组合,Circle 类包含了一个 Point 对象 center,这样 Circle 就拥有了自己的属性和方法。在 main 函数中,我们可以通过调用 getCenter 函数来获取 Circle 对象的中心坐标。
#include <cmath> #include <iostream> using namespace std; class Circle{ private: int x,y; int radius; public: Circle(int x,int y,int radius); double distance(const Circle &c); }; Circle::Circle(int x,int y,int radius):x(x),y(y),radius(radius){ } double Circle::distance(const Circle &c){ double dis=sqrt((x-c.x)*(x-c.x)+(y-c.y)*(y-c.y)); return dis; } int main(int argc, char* argv) { Circle c1(0,0,1),c2(1,1,1); cout<<"c1和c2的距离"<<endl; return 0; }输出的结果
程序中并没有输出 c1 和 c2 的距离,需要在 main 函数中调用 distance 函数并输出结果,如下所示:
```
#include <cmath>
#include <iostream>
using namespace std;
class Circle{
private:
int x,y;
int radius;
public:
Circle(int x,int y,int radius);
double distance(const Circle &c);
};
Circle::Circle(int x,int y,int radius):x(x),y(y),radius(radius){ }
double Circle::distance(const Circle &c){
double dis=sqrt((x-c.x)*(x-c.x)+(y-c.y)*(y-c.y));
return dis;
}
int main(int argc, char* argv) {
Circle c1(0,0,1),c2(1,1,1);
cout<<"c1和c2的距离为:"<<c1.distance(c2)<<endl;
return 0;
}
```
输出结果为:
```
c1和c2的距离为:1.41421
```
相关推荐
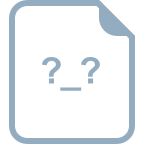













