tf实现vgg网络的代码
时间: 2023-10-26 18:11:51 浏览: 86
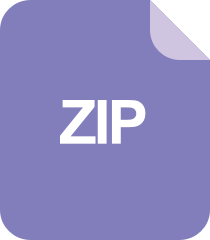
基于numpy的VGG网络实现
以下是TensorFlow实现VGG网络的代码:
```python
import tensorflow as tf
def vgg_net(inputs):
# 定义卷积层函数
def conv_layer(inputs, filters, kernel_size, strides, padding, activation):
conv = tf.layers.conv2d(inputs=inputs, filters=filters, kernel_size=kernel_size,
strides=strides, padding=padding, activation=activation)
return conv
# 定义最大池化层函数
def max_pool(inputs, pool_size, strides, padding):
pool = tf.layers.max_pooling2d(inputs=inputs, pool_size=pool_size,
strides=strides, padding=padding)
return pool
# 定义输入层
input_layer = tf.reshape(inputs, [-1, 224, 224, 3])
# 定义卷积层
conv1_1 = conv_layer(input_layer, 64, 3, 1, 'same', tf.nn.relu)
conv1_2 = conv_layer(conv1_1, 64, 3, 1, 'same', tf.nn.relu)
pool1 = max_pool(conv1_2, 2, 2, 'valid')
conv2_1 = conv_layer(pool1, 128, 3, 1, 'same', tf.nn.relu)
conv2_2 = conv_layer(conv2_1, 128, 3, 1, 'same', tf.nn.relu)
pool2 = max_pool(conv2_2, 2, 2, 'valid')
conv3_1 = conv_layer(pool2, 256, 3, 1, 'same', tf.nn.relu)
conv3_2 = conv_layer(conv3_1, 256, 3, 1, 'same', tf.nn.relu)
conv3_3 = conv_layer(conv3_2, 256, 3, 1, 'same', tf.nn.relu)
pool3 = max_pool(conv3_3, 2, 2, 'valid')
conv4_1 = conv_layer(pool3, 512, 3, 1, 'same', tf.nn.relu)
conv4_2 = conv_layer(conv4_1, 512, 3, 1, 'same', tf.nn.relu)
conv4_3 = conv_layer(conv4_2, 512, 3, 1, 'same', tf.nn.relu)
pool4 = max_pool(conv4_3, 2, 2, 'valid')
conv5_1 = conv_layer(pool4, 512, 3, 1, 'same', tf.nn.relu)
conv5_2 = conv_layer(conv5_1, 512, 3, 1, 'same', tf.nn.relu)
conv5_3 = conv_layer(conv5_2, 512, 3, 1, 'same', tf.nn.relu)
pool5 = max_pool(conv5_3, 2, 2, 'valid')
# 将卷积层输出展开
flatten = tf.layers.flatten(pool5)
# 定义全连接层
fc6 = tf.layers.dense(flatten, 4096, activation=tf.nn.relu)
fc7 = tf.layers.dense(fc6, 4096, activation=tf.nn.relu)
fc8 = tf.layers.dense(fc7, 1000, activation=None)
# 定义输出层
output = tf.nn.softmax(fc8)
return output
```
注意,该代码实现了VGG16网络的结构,最后输出1000个类别的概率。如果需要输出其他数量的类别概率,可以修改最后一层全连接层的输出大小。同时,如果需要使用VGG19网络,只需要在上述代码中增加4个卷积层和2个全连接层即可。
阅读全文
相关推荐
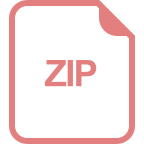
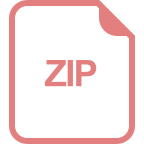
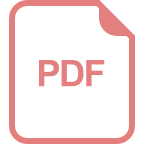



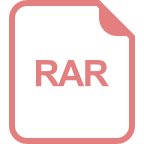
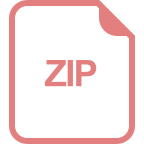
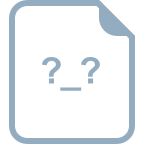
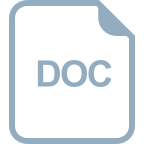
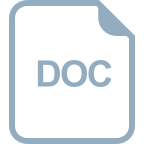
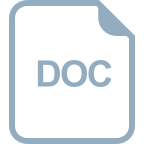




