python爬取微博关键词
时间: 2023-10-06 10:07:52 浏览: 110
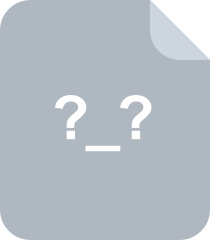
微博关键词爬取.py

要爬取微博关键词需要以下步骤:
1. 安装Python爬虫库,比如requests、beautifulsoup4、selenium等。
2. 登录微博账号,获取cookie。
3. 构造微博关键词搜索的URL,比如:https://s.weibo.com/weibo?q=关键词&Refer=q。
4. 使用requests库向该URL发送请求,并设置cookie。
5. 解析响应内容,获取微博搜索结果的页面源码。
6. 使用beautifulsoup4库解析页面源码,提取需要的信息,比如微博内容、发布时间、转发数、评论数、点赞数等。
7. 将提取的信息保存到本地文件或数据库中。
下面是一个简单的示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 设置请求头和cookie
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
cookie = 'your cookie here'
# 构造搜索URL
keyword = 'Python'
url = 'https://s.weibo.com/weibo?q=' + keyword + '&Refer=q'
# 发送请求
response = requests.get(url, headers=headers, cookies={'Cookie': cookie})
# 解析页面源码
soup = BeautifulSoup(response.text, 'html.parser')
# 提取微博信息
for card in soup.find_all(class_='card-wrap'):
content = card.find(class_='txt').text.strip()
pub_time = card.find(class_='time').text.strip()
reposts = card.find(class_='m-lev').find_all('span')[1].text.strip()
comments = card.find(class_='m-lev').find_all('span')[2].text.strip()
likes = card.find(class_='m-lev').find_all('span')[3].text.strip()
# 打印微博信息
print('微博内容:', content)
print('发布时间:', pub_time)
print('转发数:', reposts)
print('评论数:', comments)
print('点赞数:', likes)
print('------------------')
```
需要注意的是,微博有反爬虫机制,可能会出现验证码或者IP被封的情况。建议设置延时,或者使用代理IP来进行爬取。
阅读全文
相关推荐
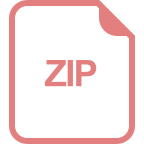




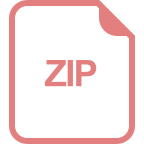
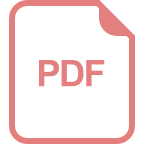
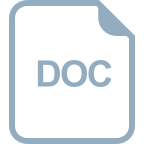







